version: 1.5 last updated: 2025-04-25 17:00
Assignment 2 - CS Amusement Park!
Overview
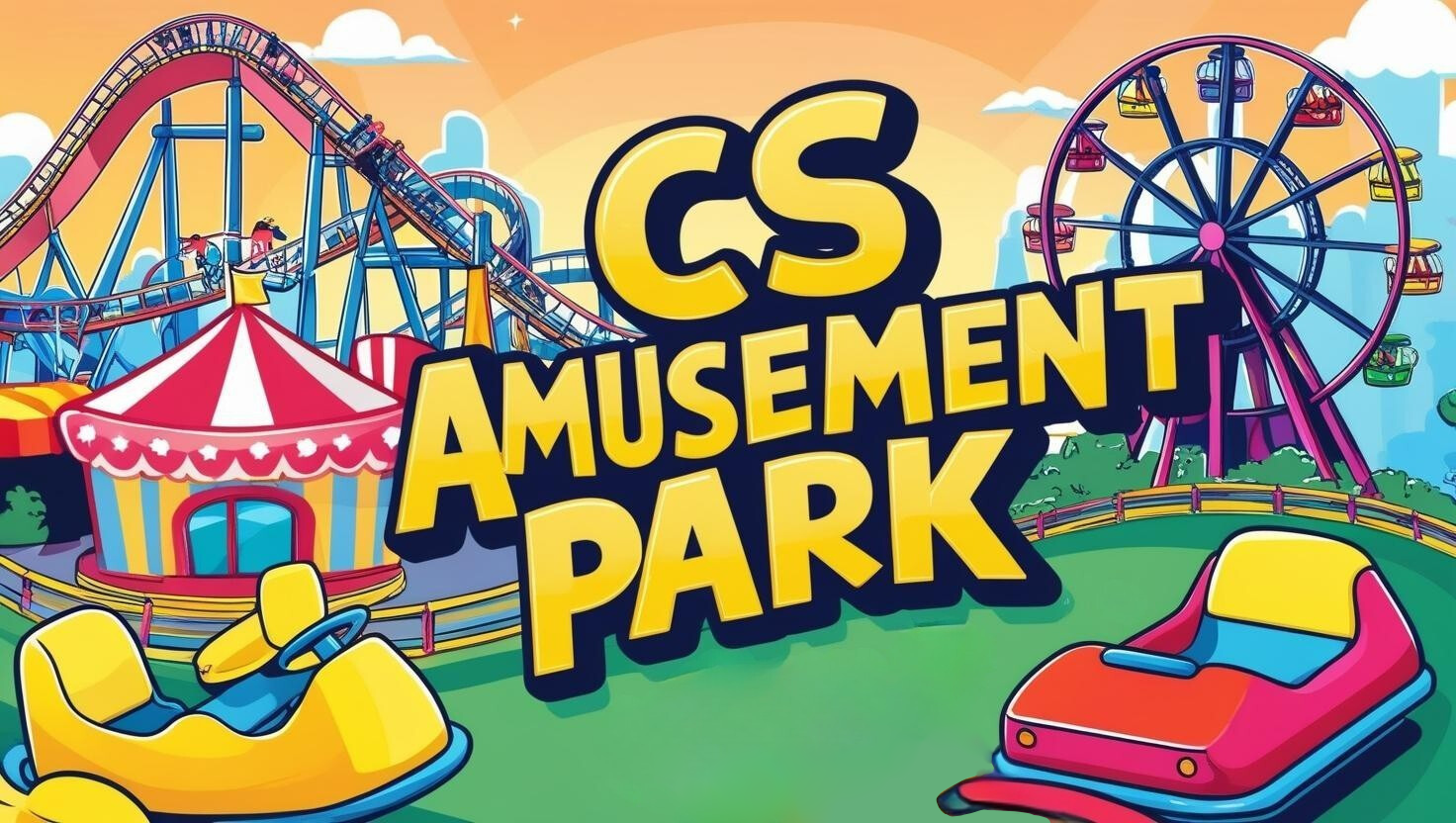
In response to a growing demand for better theme park management, the Amusement Park Association has recruited you to create a program that designs and simulates the operations of a bustling amusement park to ensure all visitors leave with a smile.
Assignment Structure
This assignment will test your ability to create, use, manipulate and solve problems using linked lists. To do this, you will be implementing an amusement park simulator, where rides are represented as a linked list, stored within the park. Each ride will contain a list of visitors currently in the queue for that ride. The park will also contain a list of visitors who are currently roaming the park, and not in the queue for a specific ride.
Starter Code
This assignment utilises a multi-file system. There are three files we use in CS Amusement Park:
- Main File (
main.c
): This file contains the main function, which serves as the entry point for the program. It is responsible for displaying the welcome message, prompting the user for the park's name, and initiating the command loop that interacts with the park.
A simple main()
function has been provided in main.c to get you started. This function outlines the basic flow of the program, which you will build upon as you implement the amusement park's functionality.
int main(void) {
// Welcome message
printf("Welcome to CS Amusement Park!\n");
printf(
" o\n"
" o |\n"
" |\n"
" . . ._._. _ .===.\n"
" |` |` ..'\\ /`.. |H| .--. .:' `:.\n"
" //\\-...-/|\\ |- o -| |H|`. /||||\\ || ||\n"
" ._.'//////,'|||`._. '`./|\\.'` |\\\\||:. .'||||||`. `:. .:'\n"
" ||||||||||||[ ]|||| /_T_\\ |:`:.--'||||||||||`--..`=:='...\n\n"
);
printf("Enter the name of the park: ");
char name[MAX_SIZE];
scan_name(name);
struct park *park = initialise_park(name);
// Command loop
command_loop(park);
// End message
printf("\nGoodbye!\n");
return 0;
}
Printing a Welcome Message
The first statement in the main()
function prints a welcome message, along with an ASCII Amusement Park. After displaying the welcome message, the program prompts the user to input a name for the amusement park.
printf("Welcome to CS Amusement Park!\n");
printf(
" o\n"
" o |\n"
" |\n"
" . . ._._. _ .===.\n"
" |` |` ..'\\ /`.. |H| .--. .:' `:.\n"
" //\\-...-/|\\ |- o -| |H|`. /||||\\ || ||\n"
" ._.'//////,'|||`._. '`./|\\.'` |\\\\||:. .'||||||`. `:. .:'\n"
" ||||||||||||[ ]|||| /_T_\\ |:`:.--'||||||||||`--..`=:='...\n\n"
);
printf("Enter the name of the park: ");
Scanning in the Park Name
In this section, the program receives input from the user for the park's name:
- It creates a character array, name, of size
MAX_SIZE
to store the park's name. - It utilises the provided
scan_name()
function, which reads the user's input and stores it in the name array.
char name[MAX_SIZE];
scan_name(name);
struct park *park = initialise_park(name);
Initialising the Park
Once the name of the park is acquired, the program proceeds to initialize the park itself:
- It calls the
initialise_park()
function, passing thename
string as an argument. This function, which you will need to implement in stage 1.1, is responsible for setting up the initial state of the park, including allocating memory and setting default values. - The function returns a pointer to a
struct park
, which represents the initialised park.
struct park *park = initialise_park(name);
Entering the Command Loop
After initialising the park, the program enters the command loop by calling the command_loop()
function which you will need to implement in stage 1.2:
- This function will handle various user commands to interact with the park, such as adding rides, managing visitors, and printing the park's status.
Ending the Program
Finally, after the user decides to quit the program, a farewell message is printed:
printf("\nGoodbye!\n");
-
Header File (
cs_amusement_park.h
): This file declares constants, enums, structs, and function prototypes for the program. You will need to add prototypes for any functions you create in this file. You should also add any of your own constants and/or enums to this file. -
Implementation File (
cs_amusement_park.c
): This file is where most of the assignment work will be done, as you will implement the logic and functionality required by the assignment here. This file also contains stubs of functions for stage 1 you to implement. It does not contain amain
function, so you will need to compile it alongsidemain.c
. You will need to define any additional functions you may need for later stages in this file.
The implementation file cs_amusement_park.c
contains some provided functions to help simplify some stages of this assignment. These functions have been fully implemented for you and should not need to be modified to complete this assignment.
These provided functions will be explained in the relevant stages of this assignment. Please read the function comments and the specification as we will suggest certain provided functions for you to use.
We have defined some structs in cs_amusement_park.h
to get you started. You may add fields to any of the structs if you wish.
struct park
- Purpose: To store all the information about the amusement park.
It contains:- The name of the park.
- The total number of visitors in the park.
- A list of rides within the park.
- A list of visitors currently roaming the park.
struct ride
- Purpose: To represent a ride within the amusement park.
It includes:- The name of the ride.
- The type of the ride (e.g., Roller Coaster, Carousel, Ferris Wheel or Bumper Cars).
- The maximum number of riders it can accommodate.
- The maximum number of visitors that can wait in the queue.
- The minimum height requirement for the ride.
- A queue of visitors waiting for the ride.
- A pointer to the next ride in the park.
struct visitor
- Purpose: To represent a visitor in the amusement park.
It contains:- The visitor's name.
- The visitor's height.
- A pointer to the next visitor in the park or in a ride queue.
The following enum definition is also provided for you in cs_amusement_park.h
. You can create your own enums if you would like, but you should not modify the provided enums.
enum ride_type
- Purpose: To represent the type of ride within the amusement park.
The possible types are:ROLLER_COASTER
,CAROUSEL
,FERRIS_WHEEL
,BUMPER_CARS
,INVALID
.
Getting Started
There are a few steps to getting started with CS Amusement Park.
- Create a new folder for your assignment work and move into it.
mkdir ass2 cd ass2
- Use this command on your CSE account to copy the file into your current directory:
1511 fetch-activity cs_amusement_park
- Run
1511 autotest cs_amusement_park
to make sure you have correctly downloaded the file.
1511 autotest cs_amusement_park
-
Read through Stage 1.
-
Spend a few minutes playing with the reference solution -- get a feel for how the assignment works.
1511 cs_amusement_park
-
Think about your solution, draw some diagrams to help you get started.
-
Start coding!
Reference Implementation
To help you understand the proper behaviour of the Amusement Park Simulator, we have provided a reference implementation. If you have any questions about the behaviour of your assignment, you can check and compare it to the reference implementation.
To run the reference implementation, use the following command:
1511 cs_amusement_park
You might want to start by running the ?
command:
1511 cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW! Enter command: ? ======================[ CS Amusement Park ]====================== ===============[ Usage Info ]=============== a r [ride_name] [ride_type] Add a ride to the park. a v [visitor_name] [visitor_height] Add a visitor to the park. i [index] [ride_name] [ride_type] Insert a ride at a specific position in the park's ride list. j [ride_name] [visitor_name] Add a visitor to the queue of a specific ride. m [visitor_name] [ride_name] Move a visitor from roaming to a ride's queue. d [visitor_name] Remove a visitor from any ride queue and return to roaming. p Print the current state of the park, including rides and visitors. t Display the total number of visitors in the park, including those roaming and in ride queues. c [start_ride] [end_ride] Count and display the number of visitors in queues between the specified start and end rides, inclusive. l [visitor_name] Remove a visitor entirely from the park. r Operate all rides, allowing visitors to enjoy the rides and moving them to roaming after their ride. M [ride_type] Merge the two smallest rides of the specified type. s [n] [ride_name] Split an existing ride into `n` smaller rides. q Quit the program and free all allocated resources. ? Show this help information. =================================================================
Allowed C Features
In this assignment, there are no restrictions on C Features, except for those in the Style Guide. If you choose to disregard this advice, you must still follow the Style Guide.
You also may be unable to get help from course staff if you use features not taught in COMP1511. Features that the Style Guide identifies as illegal will result in a penalty during marking. You can find the style marking rubric above. Please note that this assignment must be completed using only Linked Lists. You may only use strings for their intended purpose: storing names such as those of parks, rides, or visitors. This includes reading, writing, and storing these names. You must not use arrays of any kind to assist with solving the logic of the assignment. Arrays must not be used to store, manipulate, or process data beyond string names. Doing so will result in a zero for performance. All core data structures and logic must be implemented using linked lists only.
FAQ
Q: Can I edit the given starter code functions/structs/enums?
A: Yes! Feel free to modify/replace/discard any of the provided:
#define
constants,- enums,
- structs, and
- functions.
For example: you may wish to add fields to the provided struct park
definition.
You are also encouraged to create your own constants, enums, structs, and helper functions.
Q: Can I use X other C feature
A: For everything not taught in the course, check the style guide:
If the guide says "avoid", it may incur a style mark penalty if not used correctly. If the guide says "illegal" or "do not use," it will result in a style mark penalty (see the style marking rubric). These rules exist to ensure that everyone develops the required foundational knowledge and to maintain a level playing field for all students.
Q: Can I use arrays
A: No, you cannot use arrays in any part of this assignment. The only exception is when using them to store strings. Using arrays for any part of this assignment will result in a 0 for performance in this assignment.
A video explanation to help you get started with the assignment can here found here:
Stages
The assignment has been divided into incremental stages.
Stage 1
- Stage 1.1 - Creating the Park.
- Stage 1.2 - Command Loop and Help.
- Stage 1.3 - Append Rides and Visitors.
- Stage 1.4 - Printing the Park.
- Stage 1.5 - Handling Errors.
Stage 2
- Stage 2.1 - Inserting New Rides.
- Stage 2.2 - Add Visitors to the Queue of Rides.
- Stage 2.3 - Remove Visitors from the Queue of Rides.
- Stage 2.4 - Move Visitors to Different Rides.
- Stage 2.5 - Total Visitors.
Stage 3
- Stage 3.1 - End of Day.
- Stage 3.2 - Leave the Park.
- Stage 3.3 - Operate the Rides.
- Stage 3.4 - Ride Shut Down.
Stage 4
- Stage 4.1 - Merge.
- Stage 4.2 - Split.
- Stage 4.3 - Scheduled.
Extension (not for marks)
- SplashKit - Create and Share a Graphical User Interface.
Your Tasks
This assignment consists of four stages. Each stage builds on the work of the previous stage, and each stage has a higher complexity than its predecessor. You should complete the stages in order.
Stage 1
For Stage 1 of this assignment, you will be implementing some basic commands to set up your amusement park simulator! This milestone has been divided into five substages:
- Stage 1.1 - Creating the Park.
- Stage 1.2 - Command Loop and Help.
- Stage 1.3 - Append Rides and Visitors.
- Stage 1.4 - Printing the park.
- Stage 1.5 - Handling Errors.
Stage 1.1 - Creating the park
As you've probably found out by now, it can be really handy to have a function that malloc
s,
initialises, and returns a single linked list node.
So in Stage 1.1, we will be implementing a function which does exactly that for a struct park
, struct ride
and struct visitor
.
You'll find the following unimplemented function stubs in cs_amusement_park.c
, in the starter code:
// Stage 1.1
// Function to initialise the park
// Params:
// name - the name of the park
// Returns: a pointer to the park
struct park *initialise_park(char name[MAX_SIZE]) {
// TODO: Replace this with your code
printf("initialise park not yet implemented\n");
return NULL;
}
// Stage 1.1
// Function to create a visitor
// Params:
// name - the name of the visitor
// height - the height of the visitor
// Returns: a pointer to the visitor
struct visitor *create_visitor(char name[MAX_SIZE], double height) {
// TODO: Replace this with your code
printf("Create visitor not yet implemented\n");
return NULL;
}
// Stage 1.1
// Function to create a ride
// Params:
// name - the name of the ride
// type - the type of the ride
// Returns: a pointer to the ride
struct ride *create_ride(char name[MAX_SIZE], enum ride_type type) {
// TODO: Replace this with your code
printf("Create ride not yet implemented\n");
return NULL;
}
Your task is to complete these functions, so that they:
- Malloc the space required.
- Copy in all the initial data.
- Initialises all other fields to some reasonable value.
- Return the relevant struct.
When creating rides, the capacity and minimum height will be added based on the enums.
Type | Rider Capacity | Queue Capacity | Min Height (cm) |
---|---|---|---|
ROLLER_COASTER |
4 | 7 | 120 |
CAROUSEL |
6 | 9 | 60 |
FERRIS_WHEEL |
8 | 11 | 75 |
BUMPER_CARS |
10 | 13 | 100 |
Clarifications
- No error handling is required for this stage.
- The initialised park should contain a total of 0 visitors and 0 rides to begin with.
Testing
There are no autotests for Stage 1.1.
Instead, double-check your work by compiling your code using dcc
and ensure there are no warnings or errors.
You could also write some temporary testing code to check that your functions work properly when they run.
For example, you could copy the following testing code into your main function in main.c
:
#include <string.h>
#include <stdio.h>
#include "cs_amusement_park.h"
int main(void) {
// Initialise the park
char park_name[MAX_SIZE];
strcpy(park_name, "UNSW");
struct park *my_park = initialise_park(park_name);
// Print park details
printf("Park initialised:\n");
printf(" Name: %s\n", my_park->name);
printf(" Total Visitors: %d\n", my_park->total_visitors);
if (my_park->rides == NULL) {
printf(" Rides: NULL\n");
} else {
printf(" Rides not set to NULL\n");
}
if (my_park->visitors == NULL) {
printf(" Visitors: NULL\n\n");
} else {
printf(" Visitors not set to NULL\n\n");
}
// Create a ride
char ride_name[MAX_SIZE];
strcpy(ride_name, "Thunderbolt");
struct ride *roller_coaster = create_ride(ride_name, ROLLER_COASTER);
// Print ride details
printf("Ride created:\n");
printf(" Name: %s\n", roller_coaster->name);
printf(" Type: %d\n", roller_coaster->type);
printf(" Rider Capacity: %d\n", roller_coaster->rider_capacity);
printf(" Queue Capacity: %d\n", roller_coaster->queue_capacity);
printf(" Minimum Height: %.2f\n", roller_coaster->min_height);
if (roller_coaster->queue == NULL) {
printf(" Queue: NULL\n");
} else {
printf(" Queue not set to NULL\n");
}
if (roller_coaster->next == NULL) {
printf(" Next Ride: NULL\n\n");
} else {
printf(" Next Ride not set to NULL\n\n");
}
// Create a visitor
char visitor_name[MAX_SIZE];
strcpy(visitor_name, "Sasha");
struct visitor *visitor = create_visitor(visitor_name, 160.5);
// Print visitor details
printf("Visitor created:\n");
printf(" Name: %s\n", visitor->name);
printf(" Height: %.2f cm\n", visitor->height);
if (visitor->next == NULL) {
printf(" Next Visitor: NULL\n\n");
} else {
printf(" Next Visitor not set to NULL\n\n");
}
return 0;
}
This code just calls initialise_park
, create_visitor
and create_ride
to malloc
and initialise, and then prints out all of their fields.
You can compile and run your program with the following command:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park
When you run it, it should print out something like:
Park initialised: Name: UNSW Total Visitors: 0 Rides: NULL Visitors: NULL Ride created: Name: Thunderbolt Type: 0 Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00 Queue: NULL Next Ride: NULL Visitor created: Name: Sasha Height: 160.50 cm Next Visitor: NULL
Visually this can be represented as below:
Stage 1.2 - Command loop and help
Now, we'll implement the command loop, allowing your program to take in and perform different operations on the park.
You'll find the following unimplemented function stub in cs_amusement_park.c
in the starter code:
// Stage 1.2
// Function to run the command loop
//
// Params:
// park - the park
// Returns: None
void command_loop(struct park *park) {
// TODO: Replace this with your code
printf("Command loop not yet implemented\n");
return;
}
This function is already called in the main()
function in the main.c
file for you.
From this stage onwards, your program should run in a loop, scanning in and executing commands until CTRL+D
is entered.
You should implement this command loop between the welcome and goodbye messages, so that your program runs in the following order:
- First, print out the welcome message (the printf statement is included in the starter code).
- Then run in a loop until
CTRL+D
, and:
- Print out the prompt
Enter command:
- Scan in a command character
- Scan in any arguments following the command character
- Execute the command.
- After
CTRL+D
is pressed, the goodbye message should be printed (the printf statement is included in the starter code), and then your program should end.
Each command will start with a single unique character and may be followed by a variable number of arguments depending on the command.
The unique character for each different command and the number and type of the command arguments are specified in the relevant stages of this assignment.
The first command you will implement is the help command, which is described below.
Command
?
Description
When the character '?'
is entered into your program, it should run the Help command.
This command doesn't read in any arguments following the initial command and should display a message which lists
the different commands and their arguments.
The purpose of this command is to allow anyone using our program to easily look up the different commands.
A helper function: print_usage()
in cs_amusement_park.c
has been provided to help you print and format this message.
// Function to print the usage of the program
// '?' command
// Params: None
// Returns: None
void print_usage(void) {
printf(
"======================[ CS Amusement Park ]======================\n"
" ===============[ Usage Info ]=============== \n"
" a r [ride_name] [ride_type] \n"
" Add a ride to the park. \n"
" a v [visitor_name] [visitor_height] \n"
" Add a visitor to the park. \n"
" i [index] [ride_name] [ride_type] \n"
" Insert a ride at a specific position in the park's ride list.\n"
" j [ride_name] [visitor_name] \n"
" Add a visitor to the queue of a specific ride. \n"
" m [visitor_name] [ride_name] \n"
" Move a visitor from roaming to a ride's queue. \n"
" d [visitor_name] \n"
" Remove a visitor from any ride queue and return to roaming. \n"
" p \n"
" Print the current state of the park, including rides and \n"
" visitors. \n"
" t \n"
" Display the total number of visitors in the park, including \n"
" those roaming and in ride queues. \n"
" c [start_ride] [end_ride] \n"
" Count and display the number of visitors in queues between \n"
" the specified start and end rides, inclusive. \n"
" l [visitor_name] \n"
" Remove a visitor entirely from the park. \n"
" r \n"
" Operate all rides, allowing visitors to enjoy the rides \n"
" and moving them to roaming after their ride. \n"
" S [ride_name] \n"
" Shut down a ride, redistributing its visitors to other rides.\n"
" M [ride_type] \n"
" Merge the two smallest rides of the specified type. \n"
" s [n] [ride_name] \n"
" Split an existing ride into `n` smaller rides. \n"
" T [n] [command] \n"
" Schedules a command to execute in `n` ticks \n"
" q \n"
" Quit the program and free all allocated resources. \n"
" ? \n"
" Show this help information. \n"
"=================================================================\n");
}
This is what it should look like when you run the Help command:
Enter command: ? ======================[ CS Amusement Park ]====================== ===============[ Usage Info ]=============== a r [ride_name] [ride_type] Add a ride to the park. a v [visitor_name] [visitor_height] Add a visitor to the park. i [index] [ride_name] [ride_type] Insert a ride at a specific position in the park's ride list. j [ride_name] [visitor_name] Add a visitor to the queue of a specific ride. m [visitor_name] [ride_name] Move a visitor from roaming to a ride's queue. d [visitor_name] Remove a visitor from any ride queue and return to roaming. p Print the current state of the park, including rides and visitors. t Display the total number of visitors in the park, including those roaming and in ride queues. c [start_ride] [end_ride] Count and display the number of visitors in queues between the specified start and end rides, inclusive. l [visitor_name] Remove a visitor entirely from the park. r Operate all rides, allowing visitors to enjoy the rides and moving them to roaming after their ride. M [ride_type] Merge the two smallest rides of the specified type. s [n] [ride_name] Split an existing ride into `n` smaller rides. q Quit the program and free all allocated resources. ? Show this help information. =================================================================
Because of the command loop, after the Help command has finished running, your program should print out
Enter command:
and then wait for the user to either enter another command, or press CTRL+D
to end the program.
Errors
- If an invalid command is entered, your program should print
ERROR: Invalid command.
Clarifications
- All commands will begin with a single unique character.
Some will be followed by a number of arguments, depending on the command. - You can assume that commands will always be given the correct number of arguments, so you do NOT need to check for this.
Examples
Input:
UNSW [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: [CTRL+D] Goodbye!
Input:
UNSW ? [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: ? ======================[ CS Amusement Park ]====================== ===============[ Usage Info ]=============== a r [ride_name] [ride_type] Add a ride to the park. a v [visitor_name] [visitor_height] Add a visitor to the park. i [index] [ride_name] [ride_type] Insert a ride at a specific position in the park's ride list. j [ride_name] [visitor_name] Add a visitor to the queue of a specific ride. m [visitor_name] [ride_name] Move a visitor from roaming to a ride's queue. d [visitor_name] Remove a visitor from any ride queue and return to roaming. p Print the current state of the park, including rides and visitors. t Display the total number of visitors in the park, including those roaming and in ride queues. c [start_ride] [end_ride] Count and display the number of visitors in queues between the specified start and end rides, inclusive. l [visitor_name] Remove a visitor entirely from the park. r Operate all rides, allowing visitors to enjoy the rides and moving them to roaming after their ride. M [ride_type] Merge the two smallest rides of the specified type. s [n] [ride_name] Split an existing ride into `n` smaller rides. q Quit the program and free all allocated resources. T [n] [command] Scheduled the [command] to take place `n` ticks in the future. ~ [n] Progress the schedule for `n` ticks. ? Show this help information. ================================================================= Enter command: [CTRL+D] Goodbye!
Stage 1.3 - Append rides and visitors
Now it's time to start building your amusement park!
When you first run your program, the park (and your linked list representing the rides and visitors) will start empty.
It should look something like this:
To make your amusement park more interesting, we need a way of adding rides and visitors.
// Stage 1.3
// Function to add a ride to the park
// Params:
// p - the park
// Returns: None
void add_ride(struct park *park) {
// TODO: Replace this with your code
printf("Add ride not yet implemented\n");
return;
}
// Stage 1.3
// Function to add a visitor to the park
// Params:
// p - the park
// Returns: None
void add_visitor(struct park *park) {
// TODO: Replace this with your code
printf("Add visitor not yet implemented\n");
return;
}
Description
The a
command appends a ride or visitor to the park, and will be followed by another character based on whether you are appending a ride r
or a visitor v
. Then scans in more information based on that more input is entered.
If you are appending a ride, r
:
- a name and,
- a type
will also be scanned in.
If you are appending a visitor, v
:
- a name and,
- a height
will also be scanned in.
Provided helper functions
Two helper functions have been provided for this stage:
enum ride_type scan_type(void)
:- Scans the
ride_type
and returns it as anenum ride_type
. - Returns
INVALID
if theride_type
did not correspond to one of the valid ride types.
- Scans the
void scan_name(char name[MAX_SIZE])
- Scans the
name
intochar name[MAX_SIZE]
.
- Scans the
Command
a r [name] [type]
// Stage 1.3
// Function to add a ride to the park
// Params:
// p - the park
// Returns: None
void add_ride(struct park *park) {
// TODO: Replace this with your code
printf("Add ride not yet implemented\n");
return;
}
Description
When this command is run, your program should create a new ride containing the name and type, assigning all other fields based on the type, and then append it to the end of the list of rides (i.e. perform a tail insertion).
Finally, it should print out a message to confirm that the command was successful:
Ride: '[name]' added!
You should replace [name]
in this message with the name
you scanned in.
After appending one ride, your park should look something like this:
Any other rides added via this command will all be appended to the end of the list, i.e. a tail insertion, looking something like this:
Examples
Input:
UNSW a r RollerCoaster ROLLER_COASTER [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' added! Enter command: [CTRL+D] Goodbye!
Input:
UNSW a r MerryGoRound CAROUSEL a r BigWheel FERRIS_WHEEL a r SkyHigh ROLLER_COASTER [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r MerryGoRound CAROUSEL Ride: 'MerryGoRound' added! Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a r SkyHigh ROLLER_COASTER Ride: 'SkyHigh' added! Enter command: [CTRL+D] Goodbye!
Command
a v [name] [height]
// Stage 1.3
// Function to add a visitor to the park
// Params:
// p - the park
// Returns: None
void add_visitor(struct park *park) {
// TODO: Replace this with your code
printf("Add visitor not yet implemented\n");
return;
}
Description
When this command is run, your program should create a new visitor containing the name and height, and then append it to the end of the list of visitors (i.e. perform a tail insertion).
Finally, it should print out a message to confirm that the command was successful:
Visitor: '[name]' has entered the amusement park!
You should replace [name]
in this message with the name
you scanned in.
After appending one visitor, your park should look something like this:
Any other visitors added via this command will all be appended to the end of the list, i.e. a tail insertion, looking something like this:
Examples
Input:
UNSW a v Sasha 160.5 [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a v Sasha 160.5 Visitor: 'Sasha' has entered the amusement park! Enter command: [CTRL+D] Goodbye!
Input:
UNSW a v Sasha 160.5 a v Sofia 165 a v Ibby 175 a v Grace 168.2 [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a v Sasha 160.5 Visitor: 'Sasha' has entered the amusement park! Enter command: a v Sofia 165 Visitor: 'Sofia' has entered the amusement park! Enter command: a v Ibby 175 Visitor: 'Ibby' has entered the amusement park! Enter command: a v Grace 168.2 Visitor: 'Grace' has entered the amusement park! Enter command: [CTRL+D] Goodbye!
Input:
UNSW a r SwingSet CAROUSEL a v Sasha 160.5 a r SkyHigh ROLLER_COASTER a v Sofia 165 a r BigWheel FERRIS_WHEEL a v Ibby 175 a v Grace 168.2 [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r SwingSet CAROUSEL Ride: 'SwingSet' added! Enter command: a v Sasha 160.5 Visitor: 'Sasha' has entered the amusement park! Enter command: a r SkyHigh ROLLER_COASTER Ride: 'SkyHigh' added! Enter command: a v Sofia 165 Visitor: 'Sofia' has entered the amusement park! Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a v Ibby 175 Visitor: 'Ibby' has entered the amusement park! Enter command: a v Grace 168.2 Visitor: 'Grace' has entered the amusement park! Enter command: [CTRL+D] Goodbye!
Clarifications
- No error handling is required in this stage.
type
will be entered as an uppercase string and automatically converted to the correctenum ride_type
for you by thescan_type
function when the function is used.name
will always be less thanMAX_SIZE - 1
- You don't need to worry about
INVALID
until Stage 1.5.
Until then, you can assume that the returnedenum ride_type
will never beINVALID
.
Stage 1.4 - Printing the park
Command
p
// Stage 1.4
// Function to print the park
// Params:
// p - the park
// Returns: None
void print_park(struct park *park) {
// TODO: Replace this with your code
printf("Print park not yet implemented\n");
return;
}
Description
When the p
command is run, your program should print out all rides in the amusement park, from head to tail and then all the visitors, from head to tail currently roaming the park.
A function print_ride
has been provided for you to format and print a single ride:
// Function to print a ride
// Params:
// ride - the ride to print
// Returns: None
// Usage:
// ```
// print_ride(ride);
// ```
void print_ride(struct ride *ride) {
printf(" %s (%s)\n", ride->name, type_to_string(ride->type));
printf(" Rider Capacity: %d\n", ride->rider_capacity);
printf(" Queue Capacity: %d\n", ride->queue_capacity);
printf(" Minimum Height: %.2lfcm\n", ride->min_height);
printf(" Queue:\n");
struct visitor *curr_visitor = ride->queue;
if (curr_visitor == NULL) {
printf(" No visitors\n");
} else {
while (curr_visitor != NULL) {
printf(
" %s (%.2lfcm)\n", curr_visitor->name,
curr_visitor->height);
curr_visitor = curr_visitor->next;
}
}
}
Additionally, a helper function print_welcome_message
has been provided to assist you.
// Function to print a welcome message
// Params:
// name - the name of the park
// Returns: None
// Usage:
// ```
// print_welcome_message(name);
// ```
void print_welcome_message(char name[MAX_SIZE]) {
printf("===================[ %s ]===================\n", name);
}
If there are no rides in the amusement park, the following message should be printed instead:
No rides!
If there are no visitors in the amusement park, the following message should be printed instead:
No visitors!
If there are no rides and no visitors in the amusement, the following message should be printed instead:
The amusement park is empty!
Examples
Input:
UNSW a r RollerCoaster ROLLER_COASTER p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' added! Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors Visitors: No visitors! Enter command: [CTRL+D] Goodbye!
Input:
UNSW a v Sasha 160.5 p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a v Sasha 160.5 Visitor: 'Sasha' has entered the amusement park! Enter command: p ===================[ UNSW ]=================== Rides: No rides! Visitors: Sasha (160.50cm) Enter command: [CTRL+D] Goodbye!
Input:
UNSW a r SwingSet CAROUSEL a v Sasha 160.5 a r SkyHigh ROLLER_COASTER a v Sofia 165 a r BigWheel FERRIS_WHEEL a v Ibby 175 a v Grace 168.2 p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r SwingSet CAROUSEL Ride: 'SwingSet' added! Enter command: a v Sasha 160.5 Visitor: 'Sasha' has entered the amusement park! Enter command: a r SkyHigh ROLLER_COASTER Ride: 'SkyHigh' added! Enter command: a v Sofia 165 Visitor: 'Sofia' has entered the amusement park! Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a v Ibby 175 Visitor: 'Ibby' has entered the amusement park! Enter command: a v Grace 168.2 Visitor: 'Grace' has entered the amusement park! Enter command: p ===================[ UNSW ]=================== Rides: SwingSet (CAROUSEL) Rider Capacity: 6 Queue Capacity: 9 Minimum Height: 60.00cm Queue: No visitors SkyHigh (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors BigWheel (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: No visitors Visitors: Sasha (160.50cm) Sofia (165.00cm) Ibby (175.00cm) Grace (168.20cm) Enter command: [CTRL+D] Goodbye!
Stage 1.5 - Handling Errors
In this stage, you will modify your stage 1.3 code to add some restrictions.
Error conditions
- When adding a ride, if the
type
(returned by thescan_type
function) isINVALID
, the following error should be printed:
ERROR: Invalid ride type.
- When adding a ride, if there is already a ride in the park that contains the
name
, the following error should be printed.
ERROR: '[name]' already exists.
- When adding a visitor, if there is already a visitor in the park that contains the
name
, the following error should be printed.
ERROR: '[name]' already exists.
- When adding a visitor, if the height of visitor is
<
50 or>
250, the following error should be entered
ERROR: Height must be between 50 and 250.
- When adding a visitor, if the number of visitors in the park will exceed
40
, the following error should be entered
ERROR: Cannot add another visitor to the park. The park is at capacity.
.
Examples
Input:
UNSW a v Grace 45 a v Grace 450 a v Grace 160 a v Grace 170 [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a v Grace 45 ERROR: Height must be between 50 and 250. Enter command: a v Grace 450 ERROR: Height must be between 50 and 250. Enter command: a v Grace 160 Visitor: 'Grace' has entered the amusement park! Enter command: a v Grace 170 ERROR: 'Grace' already exists. Enter command: [CTRL+D] Goodbye!
Input:
UNSW a r RollerCoaster INVALID a r RollerCoaster CAROUSEL a r RollerCoaster CAROUSEL [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster INVALID ERROR: Invalid ride type. Enter command: a r RollerCoaster CAROUSEL Ride: 'RollerCoaster' added! Enter command: a r RollerCoaster CAROUSEL ERROR: 'RollerCoaster' already exists. Enter command: [CTRL+D] Goodbye!
Input:
UNSW a r RollerCoaster INVALID a r RollerCoaster CAROUSEL a v Sofia 40 a v Sasha 150 a v Grace 270 a v Ibby 160 a r MerryGoRound CAROUSEL [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster INVALID ERROR: Invalid ride type. Enter command: a r RollerCoaster CAROUSEL Ride: 'RollerCoaster' added! Enter command: a v Sofia 40 ERROR: Height must be between 50 and 250. Enter command: a v Sasha 150 Visitor: 'Sasha' has entered the amusement park! Enter command: a v Grace 270 ERROR: Height must be between 50 and 250. Enter command: a v Ibby 160 Visitor: 'Ibby' has entered the amusement park! Enter command: a r MerryGoRound CAROUSEL Ride: 'MerryGoRound' added! Enter command: [CTRL+D] Goodbye!
Clarifications
- If more than one error occurs, only the first error in the order specified above should be addressed by printing an error message. This is the same for all future commands.
- When checking if a ride or visitor name already exists in the park, the comparison is case-sensitive. For example, if a ride named
COMP1511
is already in the park, trying to add a ride namedComp1511
will not be treated as a duplicate, and no error will be raised. This is the same for all future commands.
Testing and Submission
Remember to do your own testing
Are you finished with this stage? If so, you should make sure to do the following:
- Run
1511 style
and clean up any issues a human may have reading your code. Don't forget -- 20% of your mark in the assignment is based on style and readability! - Autotest for this stage of the assignment by running the
autotest-stage
command as shown below. - Remember -- give early and give often. Only your last submission counts, but why not be safe and submit right now?
1511 style cs_amusement_park.c 1511 style cs_amusement_park.h 1511 style main.c 1511 autotest-stage 01 cs_amusement_park give cs1511 ass2_cs_amusement_park cs_amusement_park.c cs_amusement_park.h main.c
Stage 2
For Stage 2 of this assignment, you will use some more advanced linked list knowledge to modify linked list nodes, and perform calculations based on information stored over several nodes. This milestone has been divided into five substages:
- Stage 2.1 - Inserting New Rides.
- Stage 2.2 - Add Visitors to the Queue of Rides.
- Stage 2.3 - Remove Visitors from the Queue of Rides.
- Stage 2.4 - Move Visitors to Different Rides.
- Stage 2.5 - Total Visitors.
Stage 2.1 - Inserting New Rides
Currently, we can only extend our park and add new rides at the end of the list. You want to be able to customise the order of rides a little more, resulting in the need for a way to insert rides anywhere.
Command
i [n] [name] [type]
Description
The i
command is similar to a
(append ride), except that it takes in an additional integer argument n
which is the position in the park at which to insert the new ride.
It should create a new struct ride
containing the ride_name
and ride_type
and insert it into the park at position n
.
For example:
- If
n == 1
,
then the new ride should be inserted at the head of the park. - If
n == 2
,
then the new ride should be inserted after the first ride in the park. - If
n == N
,
then the new ride should be inserted after theN
th ride in the park. - If
n
is greater than the length of the park,
then the new ride should be inserted at the tail of the linked list.
After successful insertion, your program should print the following message:
Ride: '[name]' inserted!
Inserting a new ride at position n == 2
should look something like this:
Before Inserting:
After Inserting:
Errors
Just like command a
: append ride, there are restrictions on the rides that can be inserted into the park.
Specifically, if one of the following conditions is met, then the ride should NOT be inserted, and an error message should be printed out instead.
- If
n < 1
, the following error should be printed:
ERROR: n must be at least 1.
- If
type
isINVALID
, the following error should be printed:
ERROR: Invalid ride type.
- If
ride_name
already exists within the park, the following error should be printed:
ERROR: '[name]' already exists.
Examples
Input:
UNSW a r MerryGoRound CAROUSEL a r BigWheel FERRIS_WHEEL i 1 RollerCoaster ROLLER_COASTER p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r MerryGoRound CAROUSEL Ride: 'MerryGoRound' added! Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: i 1 RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' inserted! Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors MerryGoRound (CAROUSEL) Rider Capacity: 6 Queue Capacity: 9 Minimum Height: 60.00cm Queue: No visitors BigWheel (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: No visitors Visitors: No visitors! Enter command: [CTRL+D] Goodbye!
Input:
UNSW a r MerryGoRound CAROUSEL a r BigWheel FERRIS_WHEEL i 5 RollerCoaster ROLLER_COASTER p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r MerryGoRound CAROUSEL Ride: 'MerryGoRound' added! Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: i 5 RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' inserted! Enter command: p ===================[ UNSW ]=================== Rides: MerryGoRound (CAROUSEL) Rider Capacity: 6 Queue Capacity: 9 Minimum Height: 60.00cm Queue: No visitors BigWheel (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: No visitors RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors Visitors: No visitors! Enter command: [CTRL+D] Goodbye!
Input:
UNSW i 0 RollerCoaster ROLLER_COASTER p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: i 0 RollerCoaster ROLLER_COASTER ERROR: n must be at least 1. Enter command: p ===================[ UNSW ]=================== The amusement park is empty! Enter command: [CTRL+D] Goodbye!
Clarifications
- If
n
is 1, the new ride should be inserted at the head of the linked list. - If
n
is greater or equal to the length of the linked list, then the new ride should be inserted at the end of the linked list. - When checking if a ride name already exists in the park, the comparison is case-sensitive. For example, if a ride named
COMP1511
is already in the park, trying to add a ride namedComp1511
will not be treated as a duplicate, and no error will be raised.
Stage 2.2 - Add Visitors to the Queue of Rides
Now that we have built an amusement park with rides, it’s time to start letting visitors join the queues for those rides and have some fun!
Command
j [ride_name] [visitor_name]
Description
The j
command takes in two strings ride_name
and visitor_name
.
The j
command should attempt to find the ride with a name matching ride_name
, and then append the visitor currently roaming the park with name visitor_name
to its ride queue.
On success, after moving a visitor into a ride queue the program should print Visitor: '[visitor_name]' has entered the queue for '[ride_name]'.
If we have one visitor and one ride in the park, before adding the visitor to the queue for the ride the park will look something like this:
After moving the visitor into the queue for the ride with the j
command the park will look something like this:
Errors
If one of the following errors occurs, then the visitor should not join the queue of the ride, and an error message should be printed instead.
- If no ride containing
ride_name
exists, the following error should be printed:
ERROR: No ride exists with name '[ride_name]'.
- If no visitor containing
visitor_name
exists, the following error should be printed:
ERROR: No visitor exists with name '[visitor_name]'.
- If the visitor does not meet the height requirement for the ride, the following error should be printed
ERROR: '[visitor_name]' is not tall enough to ride '[ride_name]'.
- If the queue is already at capacity, the following error should be printed
ERROR: The queue for '[ride_name]' is full. '[visitor_name]' cannot join the queue.
Examples
Input:
UNSW a r BigWheel FERRIS_WHEEL a v Sofia 160 j BigWheel Sofia p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a v Sofia 160 Visitor: 'Sofia' has entered the amusement park! Enter command: j BigWheel Sofia Visitor: 'Sofia' has entered the queue for 'BigWheel'. Enter command: p ===================[ UNSW ]=================== Rides: BigWheel (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: Sofia (160.00cm) Visitors: No visitors! Enter command: [CTRL+D] Goodbye!
Input:
UNSW j RollerCoaster Sofia p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: j RollerCoaster Sofia ERROR: No ride exists with name 'RollerCoaster'. Enter command: p ===================[ UNSW ]=================== The amusement park is empty! Enter command: [CTRL+D] Goodbye!
Input:
UNSW a r BigWheel FERRIS_WHEEL a v Sasha 70 j BigWheel Sasha p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a v Sasha 70 Visitor: 'Sasha' has entered the amusement park! Enter command: j BigWheel Sasha ERROR: 'Sasha' is not tall enough to ride 'BigWheel'. Enter command: p ===================[ UNSW ]=================== Rides: BigWheel (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: No visitors Visitors: Sasha (70.00cm) Enter command: [CTRL+D] Goodbye!
Clarifications
- If more than one error occurs, only the first error in the order specified above should be addressed by printing an error message. This is the same for all future commands.
- When checking a name, case matters. For example, the name
COMP1511
, does not match the nameComp1511
. This is the same for all future commands - The
j
command only allows a visitor to enter a ride queue if they are currently roaming in the park.
Stage 2.3 - Remove Visitors from the Queue of Rides
Command
d [passenger_name]
Description
The d
command should attempt to find the visitor with name visitor_name
in a queue for a ride and remove them from the queue, returning them to the park, i.e. appending them to the tail of the park visitor list.
It should then print: Visitor: '[visitor_name]' has been removed from their ride queue and is now roaming the park.
Before removing the visitor Grace from the queue of the ride, the park will look something like this:
After running the command d Grace
the park will look something like this:
Errors
If one of the following errors occur, then the visitor should not be removed from the queue of a ride, and an error message should be printed instead.
- If no visitor containing
visitor_name
exists in a queue, the following error should be printed:
ERROR: Visitor '[visitor_name]' not found in any queue.
Examples
Input:
UNSW a r BigWheel FERRIS_WHEEL a v Sofia 160 j BigWheel Sofia d Sofia p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a v Sofia 160 Visitor: 'Sofia' has entered the amusement park! Enter command: j BigWheel Sofia Visitor: 'Sofia' has entered the queue for 'BigWheel'. Enter command: d Sofia Visitor: 'Sofia' has been removed from their ride queue and is now roaming the park. Enter command: p ===================[ UNSW ]=================== Rides: BigWheel (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: No visitors Visitors: Sofia (160.00cm) Enter command: [CTRL+D] Goodbye!
Input:
UNSW d Sasha a r BigWheel FERRIS_WHEEL a v Ibby 160 d Ibby p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: d Sasha ERROR: Visitor 'Sasha' not found in any queue. Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a v Ibby 160 Visitor: 'Ibby' has entered the amusement park! Enter command: d Ibby ERROR: Visitor 'Ibby' not found in any queue. Enter command: p ===================[ UNSW ]=================== Rides: BigWheel (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: No visitors Visitors: Ibby (160.00cm) Enter command: [CTRL+D] Goodbye!
Input:
UNSW a r BigWheel FERRIS_WHEEL a v Sofia 160 j BigWheel Sofia a v Sasha 150 a v Grace 165 a v Ibby 175 j BigWheel Grace j BigWheel Sasha p d Grace d Sofia p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a v Sofia 160 Visitor: 'Sofia' has entered the amusement park! Enter command: j BigWheel Sofia Visitor: 'Sofia' has entered the queue for 'BigWheel'. Enter command: a v Sasha 150 Visitor: 'Sasha' has entered the amusement park! Enter command: a v Grace 165 Visitor: 'Grace' has entered the amusement park! Enter command: a v Ibby 175 Visitor: 'Ibby' has entered the amusement park! Enter command: j BigWheel Grace Visitor: 'Grace' has entered the queue for 'BigWheel'. Enter command: j BigWheel Sasha Visitor: 'Sasha' has entered the queue for 'BigWheel'. Enter command: p ===================[ UNSW ]=================== Rides: BigWheel (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: Sofia (160.00cm) Grace (165.00cm) Sasha (150.00cm) Visitors: Ibby (175.00cm) Enter command: d Grace Visitor: 'Grace' has been removed from their ride queue and is now roaming the park. Enter command: d Sofia Visitor: 'Sofia' has been removed from their ride queue and is now roaming the park. Enter command: p ===================[ UNSW ]=================== Rides: BigWheel (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: Sasha (150.00cm) Visitors: Ibby (175.00cm) Grace (165.00cm) Sofia (160.00cm) Enter command: [CTRL+D] Goodbye!
Clarifications
- If more than one error occurs, only the first error in the order specified above should be addressed by printing an error message. This is the same for all future commands.
- When checking a name, case matters. For example, the name
COMP1511
, does not match the nameComp1511
. This is the same for all future commands
Stage 2.4 - Move Visitors to Different Rides
Command
m [visitor_name] [ride_name]
Description
The m
command should attempt to find the visitor in the park and move them to the queue for ride_name
.
The visitor may be roaming the park or in the queue for any ride.
On success, after moving a visitor into the ride queue the program should print Visitor: '[visitor_name]' has been moved to the queue for '[ride_name]'.
Before removing the visitor Sasha, the park will look something like this:
After running the command m Sasha BigWheel
the park will look something like this:
Errors
If one of the following errors occur, then the visitor should not be moved, and an error message should be printed instead.
- If a ride with name
ride_name
does not exist within the park, the following error should be printed:
ERROR: No ride exists with name '[ride_name]'.
- If a visitor with name
visitor_name
does not exist in the park, nor in a queue for a ride in the park, the following error should be printed:
ERROR: No visitor with name: '[visitor_name]' exists.
- If the visitor is currently in the queue for the ride they are trying to move into, the following error should be printed:
ERROR: '[visitor_name]' is already in the queue for '[ride_name]'.
. - If the visitor does not meet the height requirement for the ride, the following error should be printed
ERROR: '[visitor_name]' is not tall enough to ride '[ride_name]'.
- If the ride queue is already at capacity, the following error should be printed
ERROR: The queue for '[ride_name]' is full. '[visitor_name]' cannot join the queue.
Examples
Input:
UNSW a r BigWheel FERRIS_WHEEL a r MerryGoRound CAROUSEL a v Sofia 160 j BigWheel Sofia m Sofia MerryGoRound p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a r MerryGoRound CAROUSEL Ride: 'MerryGoRound' added! Enter command: a v Sofia 160 Visitor: 'Sofia' has entered the amusement park! Enter command: j BigWheel Sofia Visitor: 'Sofia' has entered the queue for 'BigWheel'. Enter command: m Sofia MerryGoRound Visitor: 'Sofia' has been moved to the queue for 'MerryGoRound'. Enter command: p ===================[ UNSW ]=================== Rides: BigWheel (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: No visitors MerryGoRound (CAROUSEL) Rider Capacity: 6 Queue Capacity: 9 Minimum Height: 60.00cm Queue: Sofia (160.00cm) Visitors: No visitors! Enter command: [CTRL+D] Goodbye!
Input:
UNSW a r BigWheel FERRIS_WHEEL a v Grace 160 j BigWheel Grace m Grace BigWheel [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a v Grace 160 Visitor: 'Grace' has entered the amusement park! Enter command: j BigWheel Grace Visitor: 'Grace' has entered the queue for 'BigWheel'. Enter command: m Grace BigWheel ERROR: 'Grace' is already in the queue for 'BigWheel'. Enter command: [CTRL+D] Goodbye!
Input:
UNSW a r BigWheel FERRIS_WHEEL a r MerryGoRound CAROUSEL a v Sasha 160 j BigWheel Sofia m Sasha NonExistent p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a r MerryGoRound CAROUSEL Ride: 'MerryGoRound' added! Enter command: a v Sasha 160 Visitor: 'Sasha' has entered the amusement park! Enter command: j BigWheel Sofia ERROR: No visitor exists with name 'Sofia'. Enter command: m Sasha NonExistent ERROR: No ride exists with name 'NonExistent'. Enter command: p ===================[ UNSW ]=================== Rides: BigWheel (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: No visitors MerryGoRound (CAROUSEL) Rider Capacity: 6 Queue Capacity: 9 Minimum Height: 60.00cm Queue: No visitors Visitors: Sasha (160.00cm) Enter command: [CTRL+D] Goodbye!
Clarifications
- A visitor can be moved either from a ride's queue or from roaming the park.
- Note, you may need to go back and modify your error checking for adding a visitor to the park to account for visitors in the queue of rides.
Stage 2.5 - Total Visitors
This stage involves implementing two very similar commands. You can implement them in any order.
Command 1
t
Description
Print out the total number of visitors in the park, this includes all visitors in queues and roaming the park. You should print in the following form:
Total visitors: [total_visitors] Visitors walking around: [roaming_visitors] Visitors in queues: [queued_visitors]
Examples
Input:
UNSW a v Sofia 160 a v Sasha 150 t [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a v Sofia 160 Visitor: 'Sofia' has entered the amusement park! Enter command: a v Sasha 150 Visitor: 'Sasha' has entered the amusement park! Enter command: t Total visitors: 2 Visitors walking around: 2 Visitors in queues: 0 Enter command: [CTRL+D] Goodbye!
Command 2
c [start_name] [end_name] [direction]
Description
The c
command should attempt to find the ride with ride_name
matching start_name
, and the ride with ride_name
matching end_name
.
It should then count the total number of visitors, waiting in the queue in the specified direction
.
-
If
direction
is>
it should then count the total number of visitors, from ride:start_name
to ride:end_name
, inclusive, wrapping if needed. -
If
direction
is<
it should then count the total number of visitors, from ride:end_name
to ride:start_name
, inclusive, wrapping if needed.
It should print out the final result in the following format:
- If
direction
is>
:
Total visitors from '[start_name]' to '[end_name]': [range_visitors].
- If
direction
is<
:
Total visitors from '[end_name]' to '[start_name]': [range_visitors].
Errors
- If either
start_name
orend_name
do not exist, the following error should be printed:
ERROR: One or both rides do not exist ('[start_name]' or '[end_name]').
Examples
Input:
UNSW a r BigWheel FERRIS_WHEEL a r MerryGoRound CAROUSEL a v Grace 165 a v Ibby 170 j BigWheel Grace j MerryGoRound Ibby a v Sasha 160.5 a v Sofia 162 c BigWheel MerryGoRound > t [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a r MerryGoRound CAROUSEL Ride: 'MerryGoRound' added! Enter command: a v Grace 165 Visitor: 'Grace' has entered the amusement park! Enter command: a v Ibby 170 Visitor: 'Ibby' has entered the amusement park! Enter command: j BigWheel Grace Visitor: 'Grace' has entered the queue for 'BigWheel'. Enter command: j MerryGoRound Ibby Visitor: 'Ibby' has entered the queue for 'MerryGoRound'. Enter command: a v Sasha 160.5 Visitor: 'Sasha' has entered the amusement park! Enter command: a v Sofia 162 Visitor: 'Sofia' has entered the amusement park! Enter command: c BigWheel MerryGoRound > Total visitors from 'BigWheel' to 'MerryGoRound': 2. Enter command: t Total visitors: 4 Visitors walking around: 2 Visitors in queues: 2 Enter command: [CTRL+D] Goodbye!
Input:
UNSW a r BigWheel FERRIS_WHEEL a r MerryGoRound CAROUSEL a v Grace 165 a v Ibby 170 i 1 RollerCoaster ROLLER_COASTER j BigWheel Grace j MerryGoRound Ibby a v Sasha 160.5 a v Sofia 162 c RollerCoaster MerryGoRound < t [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a r MerryGoRound CAROUSEL Ride: 'MerryGoRound' added! Enter command: a v Grace 165 Visitor: 'Grace' has entered the amusement park! Enter command: a v Ibby 170 Visitor: 'Ibby' has entered the amusement park! Enter command: i 1 RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' inserted! Enter command: j BigWheel Grace Visitor: 'Grace' has entered the queue for 'BigWheel'. Enter command: j MerryGoRound Ibby Visitor: 'Ibby' has entered the queue for 'MerryGoRound'. Enter command: a v Sasha 160.5 Visitor: 'Sasha' has entered the amusement park! Enter command: a v Sofia 162 Visitor: 'Sofia' has entered the amusement park! Enter command: c RollerCoaster MerryGoRound < Total visitors from 'MerryGoRound' to 'RollerCoaster': 1. Enter command: t Total visitors: 4 Visitors walking around: 2 Visitors in queues: 2 Enter command: [CTRL+D] Goodbye!
Clarifications
- If
start_name
is the same asend_name
, then count the queue for that particular ride only. start_name
andend_name
should be included in the range.- If both
start_name
andend_name
are invalid, thestart_name
should be printed in the error message.
Testing and Submission
Remember to do your own testing
Are you finished with this stage? If so, you should make sure to do the following:
- Run
1511 style
and clean up any issues a human may have reading your code. Don't forget -- 20% of your mark in the assignment is based on style and readability! - Autotest for this stage of the assignment by running the
autotest-stage
command as shown below. - Remember -- give early and give often. Only your last submission counts, but why not be safe and submit right now?
1511 style cs_amusement_park.c 1511 style cs_amusement_park.h 1511 style main.c 1511 autotest-stage 02 cs_amusement_park give cs1511 ass2_cs_amusement_park cs_amusement_park.c cs_amusement_park.h main.c
Stage 3
In Stage 3, you'll be doing more advanced manipulation of 2D linked lists. You will also be managing your memory usage by freeing memory and preventing memory leaks. This milestone has been divided into four substages:
- Stage 3.1 - End of Day.
- Stage 3.2 - Leave the Park.
- Stage 3.3 - Operate the Rides.
- Stage 3.4 - Ride Shut Down.
Stage 3.1 - End of Day
It is time for the park to start cleaning up and closing up for the day. This means closing all rides and having all visitors leave the park.
Command
q
Description
All malloc’d memory should be freed when the q
command is used or when the program is ended with CTRL+D
. This should also cause the program to print Goodbye!
and end with no memory leaks.
Examples
Input:
UNSW q [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: q Goodbye!
Input:
UNSW a r RollerCoaster ROLLER_COASTER a v Grace 160 [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' added! Enter command: a v Grace 160 Visitor: 'Grace' has entered the amusement park! Enter command: [CTRL+D] Goodbye!
Input:
UNSW a r BigWheel FERRIS_WHEEL a r MerryGoRound CAROUSEL a v Grace 165 a v Ibby 170 j BigWheel Grace j MerryGoRound Ibby a v Sasha 160.5 a v Sofia 162 p q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: a r MerryGoRound CAROUSEL Ride: 'MerryGoRound' added! Enter command: a v Grace 165 Visitor: 'Grace' has entered the amusement park! Enter command: a v Ibby 170 Visitor: 'Ibby' has entered the amusement park! Enter command: j BigWheel Grace Visitor: 'Grace' has entered the queue for 'BigWheel'. Enter command: j MerryGoRound Ibby Visitor: 'Ibby' has entered the queue for 'MerryGoRound'. Enter command: a v Sasha 160.5 Visitor: 'Sasha' has entered the amusement park! Enter command: a v Sofia 162 Visitor: 'Sofia' has entered the amusement park! Enter command: p ===================[ UNSW ]=================== Rides: BigWheel (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: Grace (165.00cm) MerryGoRound (CAROUSEL) Rider Capacity: 6 Queue Capacity: 9 Minimum Height: 60.00cm Queue: Ibby (170.00cm) Visitors: Sasha (160.50cm) Sofia (162.00cm) Enter command: q Goodbye!
Clarifications
- From stage 3.1 on, you should check for memory leaks by compiling with
--leak-check
, as seen in the examples below. - Autotests and marking tests from this stage onwards will check for memory leaks.
Stage 3.2 - Leave the park
Command
l [visitor_name]
Description
The l
command should attempt to find a visitor with a matching visitor_name
and remove that visitor from the park, free
ing all associated memory. Once completed the program should print Visitor: '[visitor_name]' has left the park.
Errors
- If no visitor containing
visitor_name
exists in the park or in any of the queues, then the following error should be printed:
ERROR: Visitor '[visitor_name]' not found in the park.
.
Examples
Input:
UNSW a v Grace 160 l Grace p q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a v Grace 160 Visitor: 'Grace' has entered the amusement park! Enter command: l Grace Visitor: 'Grace' has left the park. Enter command: p ===================[ UNSW ]=================== The amusement park is empty! Enter command: q Goodbye!
Input:
UNSW a r RollerCoaster ROLLER_COASTER a v Sasha 160 a v Sofia 165 a v Ibby 170 j RollerCoaster Sofia j RollerCoaster Sasha j RollerCoaster Ibby l Sasha p l Sofia p q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' added! Enter command: a v Sasha 160 Visitor: 'Sasha' has entered the amusement park! Enter command: a v Sofia 165 Visitor: 'Sofia' has entered the amusement park! Enter command: a v Ibby 170 Visitor: 'Ibby' has entered the amusement park! Enter command: j RollerCoaster Sofia Visitor: 'Sofia' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Sasha Visitor: 'Sasha' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Ibby Visitor: 'Ibby' has entered the queue for 'RollerCoaster'. Enter command: l Sasha Visitor: 'Sasha' has left the park. Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Sofia (165.00cm) Ibby (170.00cm) Visitors: No visitors! Enter command: l Sofia Visitor: 'Sofia' has left the park. Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Ibby (170.00cm) Visitors: No visitors! Enter command: q Goodbye!
Input:
UNSW a v Grace 160 l NonexistentVisitor q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a v Grace 160 Visitor: 'Grace' has entered the amusement park! Enter command: l NonexistentVisitor ERROR: Visitor 'NonexistentVisitor' not found in the park. Enter command: q Goodbye!
Clarifications
- When checking a name, case matters. For example, the name
COMP1511
, does not match the nameComp1511
. This is the same for all future commands. - The visitor may be roaming the park or in the queue for a ride.
Stage 3.3 - Operate the rides
Command
r
Description
The r
command should operate all the rides such that each ride takes on the number of people equal to its rider capacity. Once the ride is complete, they exit the ride and are no longer in the ride's queue. Instead, they return to roaming the park as general park visitors. When these visitors return to roaming, they should be added to the end of the park's roaming list in the same order they were in the ride's queue. Additionally, rides should be processed in the reverse order of how they appear in the park list.
For example, before running the r
command, the park will look something like this:
After running the command the park will look something like this:
Examples
Input:
UNSW a r RollerCoaster ROLLER_COASTER a r Carousel CAROUSEL a v Grace 160 a v Sasha 150 j RollerCoaster Grace j Carousel Sasha p r p q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park UNSW e to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' added! Enter command: a r Carousel CAROUSEL Ride: 'Carousel' added! Enter command: a v Grace 160 Visitor: 'Grace' has entered the amusement park! Enter command: a v Sasha 150 Visitor: 'Sasha' has entered the amusement park! Enter command: j RollerCoaster Grace Visitor: 'Grace' has entered the queue for 'RollerCoaster'. Enter command: j Carousel Sasha Visitor: 'Sasha' has entered the queue for 'Carousel'. Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Grace (160.00cm) Carousel (CAROUSEL) Rider Capacity: 6 Queue Capacity: 9 Minimum Height: 60.00cm Queue: Sasha (150.00cm) Visitors: No visitors! Enter command: r Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors Carousel (CAROUSEL) Rider Capacity: 6 Queue Capacity: 9 Minimum Height: 60.00cm Queue: No visitors Visitors: Sasha (150.00cm) Grace (160.00cm) Enter command: q Goodbye!
Input:
UNSW a r RollerCoaster ROLLER_COASTER a v Grace 160 a v Sasha 150 a v Sofia 165 a v Ibby 175 a v Ben 172.5 a v Tammy 152 j RollerCoaster Grace j RollerCoaster Sasha j RollerCoaster Sofia j RollerCoaster Tammy j RollerCoaster Ben j RollerCoaster Ibby p r p q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' added! Enter command: a v Grace 160 Visitor: 'Grace' has entered the amusement park! Enter command: a v Sasha 150 Visitor: 'Sasha' has entered the amusement park! Enter command: a v Sofia 165 Visitor: 'Sofia' has entered the amusement park! Enter command: a v Ibby 175 Visitor: 'Ibby' has entered the amusement park! Enter command: a v Ben 172.5 Visitor: 'Ben' has entered the amusement park! Enter command: a v Tammy 152 Visitor: 'Tammy' has entered the amusement park! Enter command: j RollerCoaster Grace Visitor: 'Grace' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Sasha Visitor: 'Sasha' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Sofia Visitor: 'Sofia' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Tammy Visitor: 'Tammy' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Ben Visitor: 'Ben' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Ibby Visitor: 'Ibby' has entered the queue for 'RollerCoaster'. Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Grace (160.00cm) Sasha (150.00cm) Sofia (165.00cm) Tammy (152.00cm) Ben (172.50cm) Ibby (175.00cm) Visitors: No visitors! Enter command: r Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Ben (172.50cm) Ibby (175.00cm) Visitors: Grace (160.00cm) Sasha (150.00cm) Sofia (165.00cm) Tammy (152.00cm) Enter command: q Goodbye!
Input:
UNSW a r RollerCoaster ROLLER_COASTER a v Grace 160 a v Sasha 150 a v Sofia 165 i 1 Carousel CAROUSEL a v Ibby 175 a v Ben 172.5 a v Tammy 152 j RollerCoaster Grace j Carousel Sasha j Carousel Sofia a v Andrew 180 j Carousel Andrew a v Angela 164 j RollerCoaster Tammy j Carousel Ben j RollerCoaster Ibby j RollerCoaster Angela p r p q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' added! Enter command: a v Grace 160 Visitor: 'Grace' has entered the amusement park! Enter command: a v Sasha 150 Visitor: 'Sasha' has entered the amusement park! Enter command: a v Sofia 165 Visitor: 'Sofia' has entered the amusement park! Enter command: i 1 Carousel CAROUSEL Ride: 'Carousel' inserted! Enter command: a v Ibby 175 Visitor: 'Ibby' has entered the amusement park! Enter command: a v Ben 172.5 Visitor: 'Ben' has entered the amusement park! Enter command: a v Tammy 152 Visitor: 'Tammy' has entered the amusement park! Enter command: j RollerCoaster Grace Visitor: 'Grace' has entered the queue for 'RollerCoaster'. Enter command: j Carousel Sasha Visitor: 'Sasha' has entered the queue for 'Carousel'. Enter command: j Carousel Sofia Visitor: 'Sofia' has entered the queue for 'Carousel'. Enter command: a v Andrew 180 Visitor: 'Andrew' has entered the amusement park! Enter command: j Carousel Andrew Visitor: 'Andrew' has entered the queue for 'Carousel'. Enter command: a v Angela 164 Visitor: 'Angela' has entered the amusement park! Enter command: j RollerCoaster Tammy Visitor: 'Tammy' has entered the queue for 'RollerCoaster'. Enter command: j Carousel Ben Visitor: 'Ben' has entered the queue for 'Carousel'. Enter command: j RollerCoaster Ibby Visitor: 'Ibby' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Angela Visitor: 'Angela' has entered the queue for 'RollerCoaster'. Enter command: p ===================[ UNSW ]=================== Rides: Carousel (CAROUSEL) Rider Capacity: 6 Queue Capacity: 9 Minimum Height: 60.00cm Queue: Sasha (150.00cm) Sofia (165.00cm) Andrew (180.00cm) Ben (172.50cm) RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Grace (160.00cm) Tammy (152.00cm) Ibby (175.00cm) Angela (164.00cm) Visitors: No visitors! Enter command: r Enter command: p ===================[ UNSW ]=================== Rides: Carousel (CAROUSEL) Rider Capacity: 6 Queue Capacity: 9 Minimum Height: 60.00cm Queue: No visitors RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors Visitors: Grace (160.00cm) Tammy (152.00cm) Ibby (175.00cm) Angela (164.00cm) Sasha (150.00cm) Sofia (165.00cm) Andrew (180.00cm) Ben (172.50cm) Enter command: q Goodbye!
Clarifications
- Rides are processed in reverse order of their appearance in the linked list, and visitors from each ride are appended to the roaming list in the same order as they appeared in the ride's queue.
- If there are no rides in the park, the
r
command should do nothing. - If a ride's queue is empty, no visitors are processed for that ride, and the ride is simply skipped.
Stage 3.4 - Ride Shut Down
Command
S [ride_name]
Description
Oh no! A ride has malfunctioned and needs to shut down for the day! The S
command should shut down the ride with the name ride_name
and move all of the visitors in the queue for that ride to the next ride(s) of the same type.
The visitors in the queue of the shutting-down ride are first added to the queue of the first available ride of the same type, filling it to its capacity.
- If there are still remaining visitors after filling the first ride, they are then transferred to the next available ride of the same type, and so on, until all visitors are moved.
- If the total capacity of the available rides of the same type is insufficient to accommodate all the visitors from the malfunctioning ride, then none of the visitors will be added to any ride queue. Instead, all visitors from the queue of the malfunctioning ride will be added back to the list of visitors roaming the park.
After handling the visitors, the malfunctioning ride must be removed from the park, and its memory freed.
For example, before a ride shutdown, the park may look something like this:
After running the command S Carousel
the park will look something like this:
Errors
- If no ride containing
ride_name
exists in the park, then the following error should be printed:
ERROR: No ride exists with name: '[ride_name]'.
- If there is not enough space to redistribute all visitors the following error should dbe printer:
ERROR: Not enough capacity to redistribute all visitors from '[ride_name]'.
Examples
Input:
UNSW a r RollerCoaster ROLLER_COASTER a r Carousel CAROUSEL a r SwingSet CAROUSEL a v Grace 160 j Carousel Grace S Carousel p q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' added! Enter command: a r Carousel CAROUSEL Ride: 'Carousel' added! Enter command: a r SwingSet CAROUSEL Ride: 'SwingSet' added! Enter command: a v Grace 160 Visitor: 'Grace' has entered the amusement park! Enter command: j Carousel Grace Visitor: 'Grace' has entered the queue for 'Carousel'. Enter command: S Carousel Ride: 'Carousel' shut down. Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors SwingSet (CAROUSEL) Rider Capacity: 6 Queue Capacity: 9 Minimum Height: 60.00cm Queue: Grace (160.00cm) Visitors: No visitors! Enter command: q Goodbye!
Input:
UNSW a r RollerCoaster ROLLER_COASTER a r Carousel CAROUSEL a v Grace 160 a v Sasha 150 j Carousel Grace j Carousel Sasha S Carousel p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' added! Enter command: a r Carousel CAROUSEL Ride: 'Carousel' added! Enter command: a v Grace 160 Visitor: 'Grace' has entered the amusement park! Enter command: a v Sasha 150 Visitor: 'Sasha' has entered the amusement park! Enter command: j Carousel Grace Visitor: 'Grace' has entered the queue for 'Carousel'. Enter command: j Carousel Sasha Visitor: 'Sasha' has entered the queue for 'Carousel'. Enter command: S Carousel ERROR: Not enough capacity to redistribute all visitors from 'Carousel'. Ride: 'Carousel' shut down. Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors Visitors: Grace (160.00cm) Sasha (150.00cm) Enter command: [CTRL+D] Goodbye!
Input:
UNSW a r RollerCoaster ROLLER_COASTER a r LoopyRoller ROLLER_COASTER a r MegaRoller ROLLER_COASTER a v Sasha 160 a v Tammy 170 a v Sofia 165 a v Grace 155 a v Ibby 150 a v Ben 145 a v Andrew 175 j RollerCoaster Sofia j RollerCoaster Andrew j RollerCoaster Sasha j RollerCoaster Ben j RollerCoaster Tammy j RollerCoaster Ibby j RollerCoaster Grace p a v Angela 164 j LoopyRoller Angela S RollerCoaster p S LoopyRoller p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' added! Enter command: a r LoopyRoller ROLLER_COASTER Ride: 'LoopyRoller' added! Enter command: a r MegaRoller ROLLER_COASTER Ride: 'MegaRoller' added! Enter command: a v Sasha 160 Visitor: 'Sasha' has entered the amusement park! Enter command: a v Tammy 170 Visitor: 'Tammy' has entered the amusement park! Enter command: a v Sofia 165 Visitor: 'Sofia' has entered the amusement park! Enter command: a v Grace 155 Visitor: 'Grace' has entered the amusement park! Enter command: a v Ibby 150 Visitor: 'Ibby' has entered the amusement park! Enter command: a v Ben 145 Visitor: 'Ben' has entered the amusement park! Enter command: a v Andrew 175 Visitor: 'Andrew' has entered the amusement park! Enter command: j RollerCoaster Sofia Visitor: 'Sofia' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Andrew Visitor: 'Andrew' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Sasha Visitor: 'Sasha' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Ben Visitor: 'Ben' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Tammy Visitor: 'Tammy' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Ibby Visitor: 'Ibby' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Grace Visitor: 'Grace' has entered the queue for 'RollerCoaster'. Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Sofia (165.00cm) Andrew (175.00cm) Sasha (160.00cm) Ben (145.00cm) Tammy (170.00cm) Ibby (150.00cm) Grace (155.00cm) LoopyRoller (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors MegaRoller (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors Visitors: No visitors! Enter command: a v Angela 164 Visitor: 'Angela' has entered the amusement park! Enter command: j LoopyRoller Angela Visitor: 'Angela' has entered the queue for 'LoopyRoller'. Enter command: S RollerCoaster Ride: 'RollerCoaster' shut down. Enter command: p ===================[ UNSW ]=================== Rides: LoopyRoller (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Angela (164.00cm) Sofia (165.00cm) Andrew (175.00cm) Sasha (160.00cm) Ben (145.00cm) Tammy (170.00cm) Ibby (150.00cm) MegaRoller (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Grace (155.00cm) Visitors: No visitors! Enter command: S LoopyRoller ERROR: Not enough capacity to redistribute all visitors from 'LoopyRoller'. Ride: 'LoopyRoller' shut down. Enter command: p ===================[ UNSW ]=================== Rides: MegaRoller (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Grace (155.00cm) Visitors: Angela (164.00cm) Sofia (165.00cm) Andrew (175.00cm) Sasha (160.00cm) Ben (145.00cm) Tammy (170.00cm) Ibby (150.00cm) Enter command: [CTRL+D] Goodbye!
Clarifications
- After the visitors from the malfunctioning ride have been successfully transferred, that ride must be removed from the park and its memory
free
'd. - If a ride's queue is already at full capacity, no additional visitors should be added to it.
- The available rides of the same type should be processed in the order they appear in the park.
- Visitors are moved to the first ride until it reaches capacity, then overflow to the next, and so on.
- If there aren't enough rides of the same type to accommodate all visitors affected by a ride shut down, none will be added to any ride queues. Instead, they'll return to the roaming visitor list in their original order.
Testing and Submission
Remember to do your own testing
Are you finished with this stage? If so, you should make sure to do the following:
- Run
1511 style
and clean up any issues a human may have reading your code. Don't forget -- 20% of your mark in the assignment is based on style and readability! - Autotest for this stage of the assignment by running the
autotest-stage
command as shown below. - Remember -- give early and give often. Only your last submission counts, but why not be safe and submit right now?
1511 style cs_amusement_park.c 1511 style cs_amusement_park.h 1511 style main.c 1511 autotest-stage 03 cs_amusement_park give cs1511 ass2_cs_amusement_park cs_amusement_park.c cs_amusement_park.h main.c
Stage 4
This stage is for students who want to challenge themselves, and solve more complicated linked lists and programming problems. This milestone has been divided into three substages:
- Stage 4.1 - Merge.
- Stage 4.2 - Split.
- Stage 4.3 - Scheduled.
Stage 4.1 - Merge
Command
M [ride_type]
Description
Merge the two rides of the given ride_type
that have the smallest queue lengths into a single larger ride, combining their queues and capacities. The visitor queues from both rides should be merged alternately into the smaller ride, starting with the queue of the ride that has the longer queue, while the other ride is removed. If the queues are the same length, start with the ride that appears first, closest to the front of the park, in the list of rides. In this case the ride whose closer to the head of the park remains in the park, while the other ride is removed.
On success the program should print Merged the two smallest rides of type '[ride_type]'.
.
Errors
- If there is not enough rides, i.e. 2 or more rides of the same type in the park, the following error should be printed:
ERROR: Not enough rides of the specified type to merge.
Examples
Input:
UNSW a r RollerCoaster_1 ROLLER_COASTER a r RollerCoaster_2 ROLLER_COASTER a v Sasha 150 a v Sofia 160 a v Grace 155 a v Ibby 165 j RollerCoaster_1 Sasha j RollerCoaster_1 Sofia j RollerCoaster_2 Grace j RollerCoaster_2 Ibby p M ROLLER_COASTER p q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster_1 ROLLER_COASTER Ride: 'RollerCoaster_1' added! Enter command: a r RollerCoaster_2 ROLLER_COASTER Ride: 'RollerCoaster_2' added! Enter command: a v Sasha 150 Visitor: 'Sasha' has entered the amusement park! Enter command: a v Sofia 160 Visitor: 'Sofia' has entered the amusement park! Enter command: a v Grace 155 Visitor: 'Grace' has entered the amusement park! Enter command: a v Ibby 165 Visitor: 'Ibby' has entered the amusement park! Enter command: j RollerCoaster_1 Sasha Visitor: 'Sasha' has entered the queue for 'RollerCoaster_1'. Enter command: j RollerCoaster_1 Sofia Visitor: 'Sofia' has entered the queue for 'RollerCoaster_1'. Enter command: j RollerCoaster_2 Grace Visitor: 'Grace' has entered the queue for 'RollerCoaster_2'. Enter command: j RollerCoaster_2 Ibby Visitor: 'Ibby' has entered the queue for 'RollerCoaster_2'. Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster_1 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Sasha (150.00cm) Sofia (160.00cm) RollerCoaster_2 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Grace (155.00cm) Ibby (165.00cm) Visitors: No visitors! Enter command: M ROLLER_COASTER Merged the two smallest rides of type 'ROLLER_COASTER'. Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster_1 (ROLLER_COASTER) Rider Capacity: 8 Queue Capacity: 14 Minimum Height: 120.00cm Queue: Sasha (150.00cm) Grace (155.00cm) Sofia (160.00cm) Ibby (165.00cm) Visitors: No visitors! Enter command: q Goodbye!
Input:
UNSW a r Carousel_1 CAROUSEL p M CAROUSEL p q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r Carousel_1 CAROUSEL Ride: 'Carousel_1' added! Enter command: p ===================[ UNSW ]=================== Rides: Carousel_1 (CAROUSEL) Rider Capacity: 6 Queue Capacity: 9 Minimum Height: 60.00cm Queue: No visitors Visitors: No visitors! Enter command: M CAROUSEL ERROR: Not enough rides of the specified type to merge. Enter command: p ===================[ UNSW ]=================== Rides: Carousel_1 (CAROUSEL) Rider Capacity: 6 Queue Capacity: 9 Minimum Height: 60.00cm Queue: No visitors Visitors: No visitors! Enter command: q Goodbye!
Input:
UNSW a r FerrisWheel_1 FERRIS_WHEEL a r FerrisWheel_2 FERRIS_WHEEL a v Sofia 155 a v Grace 165 a v Ibby 170 j FerrisWheel_1 Grace j FerrisWheel_1 Sofia j FerrisWheel_2 Ibby p M FERRIS_WHEEL p q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r FerrisWheel_1 FERRIS_WHEEL Ride: 'FerrisWheel_1' added! Enter command: a r FerrisWheel_2 FERRIS_WHEEL Ride: 'FerrisWheel_2' added! Enter command: a v Sofia 155 Visitor: 'Sofia' has entered the amusement park! Enter command: a v Grace 165 Visitor: 'Grace' has entered the amusement park! Enter command: a v Ibby 170 Visitor: 'Ibby' has entered the amusement park! Enter command: j FerrisWheel_1 Grace Visitor: 'Grace' has entered the queue for 'FerrisWheel_1'. Enter command: j FerrisWheel_1 Sofia Visitor: 'Sofia' has entered the queue for 'FerrisWheel_1'. Enter command: j FerrisWheel_2 Ibby Visitor: 'Ibby' has entered the queue for 'FerrisWheel_2'. Enter command: p ===================[ UNSW ]=================== Rides: FerrisWheel_1 (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: Grace (165.00cm) Sofia (155.00cm) FerrisWheel_2 (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: Ibby (170.00cm) Visitors: No visitors! Enter command: M FERRIS_WHEEL Merged the two smallest rides of type 'FERRIS_WHEEL'. Enter command: p ===================[ UNSW ]=================== Rides: FerrisWheel_2 (FERRIS_WHEEL) Rider Capacity: 16 Queue Capacity: 22 Minimum Height: 75.00cm Queue: Grace (165.00cm) Ibby (170.00cm) Sofia (155.00cm) Visitors: No visitors! Enter command: q Goodbye!
Clarifications
- If there are no two rides of the
type
, then theM
command should do nothing. - Any remaining visitors from the longer queue should be appended to the end of the merged queue, in the same order in which they were in the original queue.
- The visitor queues from both rides should be merged alternately into the smaller ride, starting with the queue of the ride that has the longer queue.
- If the queues are the same length, the first ride in the list should start the combined queue and be preserved.
- The merged queue should be assigned to the ride that appears first in the list of rides in the park (i.e., the one closer to the head of the list).
Stage 4.2 - Split
Command
s [n] [ride_name]
Description
The s
command enables splitting an existing ride into multiple smaller rides, each with a portion of the original queue of visitors. The command takes two arguments:
n
: The number of new rides to create.ride_name
: The name of the original ride to be split.
It should then replace the ride with the name ride_name
, in place, with n
new rides. These new rides should be named sequentially as name_1, name_2, ..., name_n
with the suffix _i
. The queue of visitors originally in the queue of the ride being split is then divided among the n
new rides. The division should aim to distribute the visitors as evenly as possible, ensuring a fair allocation.
- If the number of visitors does not divide evenly, some rides may have one more visitor than others, starting from the first of the new split rides in sequential order.
- If the original ride name is too long to accommodate the
_i
suffix within the maximum allowed length for ride names, it should be truncated to make space for the suffix.
On success the program should print Ride '[ride_name]' split into [n] new rides.
.
Errors
- If no ride containing
ride_name
exists in the park, then the following error should be printed:
ERROR: No ride exists with name: '[ride_name]'.
- If
n <= 1
, then the following error should be printed:
ERROR: Cannot split '[ride_name]' into [n] rides. n must be > 1.
Examples
Input:
UNSW a r RollerCoaster ROLLER_COASTER a v Sasha 150 a v Grace 160 a v Sofia 155 a v Andrew 165 a v Ibby 170 j RollerCoaster Sasha j RollerCoaster Sofia j RollerCoaster Andrew j RollerCoaster Grace j RollerCoaster Ibby p s 3 RollerCoaster p q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r RollerCoaster ROLLER_COASTER Ride: 'RollerCoaster' added! Enter command: a v Sasha 150 Visitor: 'Sasha' has entered the amusement park! Enter command: a v Grace 160 Visitor: 'Grace' has entered the amusement park! Enter command: a v Sofia 155 Visitor: 'Sofia' has entered the amusement park! Enter command: a v Andrew 165 Visitor: 'Andrew' has entered the amusement park! Enter command: a v Ibby 170 Visitor: 'Ibby' has entered the amusement park! Enter command: j RollerCoaster Sasha Visitor: 'Sasha' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Sofia Visitor: 'Sofia' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Andrew Visitor: 'Andrew' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Grace Visitor: 'Grace' has entered the queue for 'RollerCoaster'. Enter command: j RollerCoaster Ibby Visitor: 'Ibby' has entered the queue for 'RollerCoaster'. Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Sasha (150.00cm) Sofia (155.00cm) Andrew (165.00cm) Grace (160.00cm) Ibby (170.00cm) Visitors: No visitors! Enter command: s 3 RollerCoaster Ride 'RollerCoaster' split into 3 new rides. Enter command: p ===================[ UNSW ]=================== Rides: RollerCoaster_1 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Sasha (150.00cm) Sofia (155.00cm) RollerCoaster_2 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Andrew (165.00cm) Grace (160.00cm) RollerCoaster_3 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Ibby (170.00cm) Visitors: No visitors! Enter command: q Goodbye!
Input:
UNSW a r test_1 ROLLER_COASTER a r test_3 ROLLER_COASTER a r test ROLLER_COASTER a v Sasha 150 a v Andrew 160 a v Tammy 155 a v Ben 165 j test Sasha j test Andrew j test Tammy j test Ben p s 3 test p q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r test_1 ROLLER_COASTER Ride: 'test_1' added! Enter command: a r test_3 ROLLER_COASTER Ride: 'test_3' added! Enter command: a r test ROLLER_COASTER Ride: 'test' added! Enter command: a v Sasha 150 Visitor: 'Sasha' has entered the amusement park! Enter command: a v Andrew 160 Visitor: 'Andrew' has entered the amusement park! Enter command: a v Tammy 155 Visitor: 'Tammy' has entered the amusement park! Enter command: a v Ben 165 Visitor: 'Ben' has entered the amusement park! Enter command: j test Sasha Visitor: 'Sasha' has entered the queue for 'test'. Enter command: j test Andrew Visitor: 'Andrew' has entered the queue for 'test'. Enter command: j test Tammy Visitor: 'Tammy' has entered the queue for 'test'. Enter command: j test Ben Visitor: 'Ben' has entered the queue for 'test'. Enter command: p ===================[ UNSW ]=================== Rides: test_1 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors test_3 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors test (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Sasha (150.00cm) Andrew (160.00cm) Tammy (155.00cm) Ben (165.00cm) Visitors: No visitors! Enter command: s 3 test Ride 'test' split into 3 new rides. Enter command: p ===================[ UNSW ]=================== Rides: test_1 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors test_3 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors test_2 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Sasha (150.00cm) Andrew (160.00cm) test_4 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Tammy (155.00cm) test_5 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: Ben (165.00cm) Visitors: No visitors! Enter command: q Goodbye!
Input:
UNSW a r a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_len ROLLER_COASTER p s 3 a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_len p s 5 a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_l_2 p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_len ROLLER_COASTER Ride: 'a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_len' added! Enter command: p ===================[ UNSW ]=================== Rides: a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_len (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors Visitors: No visitors! Enter command: s 3 a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_len Ride 'a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_len' split into 3 new rides. Enter command: p ===================[ UNSW ]=================== Rides: a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_l_1 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_l_2 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_l_3 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors Visitors: No visitors! Enter command: s 5 a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_l_2 Ride 'a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_l_2' split into 5 new rides. Enter command: p ===================[ UNSW ]=================== Rides: a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_l_1 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_l_4 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_l_5 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_l_6 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_l_7 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_l_8 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors a_very_long_string_that_will_need_to_be_sliced_when_running_the_split_command_to_fit_in_the_max_l_3 (ROLLER_COASTER) Rider Capacity: 4 Queue Capacity: 7 Minimum Height: 120.00cm Queue: No visitors Visitors: No visitors! Enter command: [CTRL+D] Goodbye!
Clarifications
- The division should aim to distribute the visitors as evenly as possible.
- If the original ride name is too long to accommodate the
_i
suffix within the maximum allowed length for ride names, it should be truncated to make space for the suffix. - If some of the sequentially numbered ride names already exist in the park, the new rides should be given the next available sequential names that do not conflict with existing ride names.
- The upperbound of
n
is the maximum size of an integer in c. - If the number of visitors does not divide evenly, some rides may have one more visitor than others, starting from the head of the list of rides in the park.
Stage 4.3 - Scheduled
For this sub-stage you will be implementing two commands:
T [n] [command]
~ [n]
Command 1
T [n] [command]
Description
The T
command schedules a command to run n
ticks into the future. Once scheduled, it will execute automatically when the simulation advances by n
number of ticks. The command takes two arguments:
n
: The number of ticks from the current moment after which the command should execute.[command]
: Any valid command that could normally be issued in the program.
Scheduling with T
is instantaneous and does not advance the simulation. Scheduled commands are stored and tracked until their countdown reaches zero and only then should they execute.
At each tick, all scheduled commands whose countdowns reach zero are executed before processing any manual commands entered on that tick. If multiple commands are scheduled for the same tick, they execute in the order they were scheduled.
Note, time in the simulation advances by one tick when any command other than T
and ~
, such as a v Sofia 160
is entered.
On success, the program should print Command scheduled to run in [n] ticks.
.
Command 2
~ [n]
Description
The ~
command moves the schedule forward by a single unit of time, also referred to as a tick. The command takes one argument n
which is the number of ticks the program should progress by.
The ~
command itself does not progress time by 1 tick, like entering a command such as a v Sofia 160
would. Instead it progresses time by n
numbers of ticks.
Errors
- If
n < 1
, then the following error should be printed:
ERROR: Invalid tick delay: [n]. Must be > 0.
- If the quit command
q
is scheduled, the following error should printed only when it is executed, not when scheduled:
ERROR: Cannot schedule quit command.
- If the scheduled
[command]
is invalid, the error is printed only when it is executed, not when scheduled.
Examples
Input:
UNSW T 2 a v Grace 150 a v Sofia 160 a v Sasha 160 p q
Input and Output:
dcc cs_amusement_park.c main.c --leak-check -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: T 2 a v Grace 150 Command scheduled to run in 2 ticks. Enter command: a v Sofia 160 Visitor: 'Sofia' has entered the amusement park! Enter command: a v Sasha 160 Visitor: 'Grace' has entered the amusement park! Visitor: 'Sasha' has entered the amusement park! Enter command: p ===================[ UNSW ]=================== Rides: No rides! Visitors: Sofia (160.00cm) Grace (150.00cm) Sasha (160.00cm) Enter command: q Goodbye!
Input:
UNSW a r BigWheel FERRIS_WHEEL T 2 s 2 BigWheel ~ 2 p T 5 M FERRIS_WHEEL a r One FERRIS_WHEEL a r Two FERRIS_WHEEL ~ 3 p [CTRL+D]
Input and Output:
dcc cs_amusement_park.c main.c -o cs_amusement_park ./cs_amusement_park Welcome to CS Amusement Park! o o | | . . ._._. _ .===. |` |` ..'\ /`.. |H| .--. .:' `:. //\-...-/|\ |- o -| |H|`. /||||\ || || ._.'//////,'|||`._. '`./|\.'` |\\||:. .'||||||`. `:. .:' ||||||||||||[ ]|||| /_T_\ |:`:.--'||||||||||`--..`=:='... Enter the name of the park: UNSW Enter command: a r BigWheel FERRIS_WHEEL Ride: 'BigWheel' added! Enter command: T 2 s 2 BigWheel Command scheduled to run in 2 ticks. Enter command: ~ 2 Ride 'BigWheel' split into 2 new rides. Enter command: p ===================[ UNSW ]=================== Rides: BigWheel_1 (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: No visitors BigWheel_2 (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: No visitors Visitors: No visitors! Enter command: T 5 M FERRIS_WHEEL Command scheduled to run in 5 ticks. Enter command: a r One FERRIS_WHEEL Ride: 'One' added! Enter command: a r Two FERRIS_WHEEL Ride: 'Two' added! Enter command: ~ 3 Merged the two smallest rides of type 'FERRIS_WHEEL'. Enter command: p ===================[ UNSW ]=================== Rides: BigWheel_1 (FERRIS_WHEEL) Rider Capacity: 16 Queue Capacity: 22 Minimum Height: 75.00cm Queue: No visitors One (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: No visitors Two (FERRIS_WHEEL) Rider Capacity: 8 Queue Capacity: 11 Minimum Height: 75.00cm Queue: No visitors Visitors: No visitors! Enter command: [CTRL+D] Goodbye!
Clarifications
n
is the number of ticks from the current moment after which the command should be executed.- The upperbound of
n
is the maximum size of an integer in c. [command]
is any valid command that would normally be issued at that moment in time.- Scheduled commands are automatically executed once their tick countdown reaches zero.
- Commands scheduled for the same tick are resolved in the order they were scheduled.
T
itself does not consume a tick. Scheduling is instantaneous and does not advance the simulation. However, any other command or an explicit tick-advance action, the~
command moves the simulation forward.- Scheduled commands resolve before processing any "manual" command issued on that tick.
- You may assume that you cannot schedule the
T
or~
commands, and these cases do not need to be handled, nor will be tested.
Testing and Submission
Remember to do your own testing
Are you finished with this stage? If so, you should make sure to do the following:
- Run
1511 style
and clean up any issues a human may have reading your code. Don't forget -- 20% of your mark in the assignment is based on style and readability! - Autotest for this stage of the assignment by running the
autotest-stage
command as shown below. - Remember -- give early and give often. Only your last submission counts, but why not be safe and submit right now?
1511 style cs_amusement_park.c 1511 style cs_amusement_park.h 1511 style main.c 1511 autotest-stage 04 cs_amusement_park give cs1511 ass2_cs_amusement_park cs_amusement_park.c cs_amusement_park.h main.c
Extension
As an extension, we have set up a starting point to add a texture pack to your game via SplashKit.
Extension activities are NOT worth any marks, nor are there any autotests. They are just for fun!
Getting Started
To get started with SplashKit, complete the SplashKit activities.
Sample Code
First, navigate to your SplashKit directory.
cd splashkit
Create a new directory for your project and cd
into it.
mkdir cs_amusement_park cd cs_amusement_park
Set up your directory for SplashKit with:
1511 setup-splashkit-directory
Run this cp
command to retrieve the sample code.
cp -n /web/cs1511/25T1/activities/cs_amusement_park/splashkit_example.c .
This should add the splashkit_example.c
sample code to your current directory.
Check that SplashKit Works
Let's check that SplashKit works by running a test file. It should display a white square with a grid inside.
skm clang++ splashkit_example.c -o splashkit_example ./splashkit_example
Have a look inside of splashkit_example.c
and note that:
- At the very top, the the SplashKit library
"splashkit.h"
is included. If you see an error here, check SplashKit #1 - Getting Started for a solution to set up your VS Code project. - In the
main()
function:open_window("SplashKit Example", 600, 400)
creates a 600×400 window for displaying graphics.- The
while (!window_close_requested(w))
loop keeps running until the user closes the window. Within this loop:process_events()
handles input or window-related actions (like closing the window).refresh_screen()
updates the window so any graphical changes (like drawing a rectangle) are displayed to the user.
Running Your SplashKit Code
When creating your SplashKit program, give it a distinctive name like splashkit_cs_amusement_park.c
.
To compile and run your program, use:
skm clang++ splashkit_cs_amusement_park.c -o splashkit_cs_amusement_park ./splashkit_cs_amusement_park
Writing Your SplashKit Program
Now that you've seen how the sample code works, it’s time to create your own SplashKit program.
If you're not sure where to begin, start by researching the SplashKit Library. The goal at first is not to understand and memorise the entire library. Rather, it's better to skim through the pages to build a broad understanding of the capabilities that SplashKit can bring to your creations.
The Graphics page is a good place to start, and the following sections will be useful:
- Windows for opening, closing, and controlling the size of windows.
- Color for using different colours.
- Graphics for drawing shapes, images, and text.
- Geometry for handling shapes such as rectangles, circles, lines, and points.
- Input for capturing and handling user input such as keyboard and mouse events.
Sample Assets
If you wish to use assets similar to the demo project, copy them to your current directory with:
cp -rn /web/cs1511/25T1/activities/cs_amusement_park/splashkit_assets .
SplashKit Gallery
Have you built something fun or interesting using SplashKit? Show it off and inspire your classmates!
Share any screenshots or short videos of your running program in the SplashKit Gallery on the Course Forum. Feel free to add a brief description or reflect on how you made it, so we can all learn from your mistakes journey 😅.
Tools
Creating Parks in Separate Files
If you are getting sick of typing in your inputs for a park every single time you run CS Amusement Park, you might want to store your input in a separate file. This allows you to see the result of the setup phase or to play your dungeon straight away.
1. Create a file for your input.
First, let's create a file to store in the input for a park that you have created. This isn't a .c
file, it's just a regular plain text file, the file extension .in
works nicely for this!
Let's put it in the same directory as your assignment code.
cd ass2 ls cs_amusement_park.c cs_amusement_park.h main.c cs_amusement_park my_park.in
2. Add your input to the file
Inside of this file, add the input for the park.
Your file could look a bit like this (including the newline at the end):
UNSW a r BigWheel FERRIS_WHEEL a v Sofia 160 a v Sasha 150 a v Grace 165 a v Ibby 175 i 1 MerryGoRound CAROUSEL p
3. Run the code with your file
Next, instead of just running ./cs_amusement_park
, lets tell the computer to first read from the file we created.
cat my_park.in - | ./cs_amusement_park
This will also work on the reference implementation too!
cat my_park.in - | 1511 cs_amusement_park
Assessment
Assignment Conditions
-
Joint work is not permitted on this assignment.
This is an individual assignment.
The work you submit must be entirely your own work. Submission of any work even partly written by any other person is not permitted.
The only exception being if you use small amounts (< 10 lines) of general purpose code (not specific to the assignment) obtained from a site such as Stack Overflow or other publicly available resources. You should attribute the source of this code clearly in an accompanying comment.
Assignment submissions will be examined, both automatically and manually for work written by others.
Do not request help from anyone other than the teaching staff of COMP1511.
Do not post your assignment code to the course forum - the teaching staff can view assignment code you have recently autotested or submitted with give.
Rationale: this assignment is an individual piece of work. It is designed to develop the skills needed to produce an entire working program. Using code written by or taken from other people will stop you learning these skills.
-
The use of code-synthesis tools, such as GitHub Copilot, is not permitted on this assignment.
The use of Generative AI to generate code solutions is not permitted on this assignment.
Rationale: this assignment is intended to develop your understanding of basic concepts. Using synthesis tools will stop you learning these fundamental concepts.
-
Sharing, publishing, distributing your assignment work is not permitted.
Do not provide or show your assignment work to any other person, other than the teaching staff of COMP1511. For example, do not share your work with friends.
Do not publish your assignment code via the internet. For example, do not place your assignment in a public GitHub repository.
Rationale: by publishing or sharing your work you are facilitating other students to use your work, which is not permitted. If they submit your work, you may become involved in an academic integrity investigation.
-
Sharing, publishing, distributing your assignment work after the completion of COMP1511 is not permitted.
For example, do not place your assignment in a public GitHub repository after COMP1511 is over.
Rationale:COMP1511 sometimes reuses assignment themes, using similar concepts and content. If students in future terms can find your code and use it, which is not permitted, you may become involved in an academic integrity investigation.
Violation of the above conditions may result in an academic integrity investigation with possible penalties, up to and including a mark of 0 in COMP1511 and exclusion from UNSW.
Relevant scholarship authorities will be informed if students holding scholarships are involved in an incident of plagiarism or other misconduct. If you knowingly provide or show your assignment work to another person for any reason, and work derived from it is submitted - you may be penalised, even if the work was submitted without your knowledge or consent. This may apply even if your work is submitted by a third party unknown to you.
If you have not shared your assignment, you will not be penalised if your work is taken without your consent or knowledge.
For more information, read the UNSW Student Code , or contact the course account. The following penalties apply to your total mark for plagiarism:
0 for the assignment | Knowingly providing your work to anyone and it is subsequently submitted (by anyone). |
0 for the assignment | Submitting any other person's work. This includes joint work. |
0 FL for COMP1511 | Paying another person to complete work. Submitting another person's work without their consent. |
Submission of Work
You should submit intermediate versions of your assignment. Every time you
autotest or submit, a copy will be saved as a backup. You can find those
backups
here
, by logging in, and choosing the yellow button next to
ass2_cs_amusement_park
.
Every time you work on the assignment and make some progress, you should copy
your work to your CSE account and submit it using the give
command below.
It is fine if intermediate versions do not compile or otherwise fail submission tests.
Only the final submitted version of your assignment will be marked.
You submit your work like this:
give cs1511 ass2_cs_amusement_park cs_amusement_park.c cs_amusement_park.h main.c
Assessment Scheme
This assignment will contribute 25% to your final mark.
80% of the marks for this assignment will be based on the performance of the
code you write in cs_amusement_park.c cs_amusement_park.h main.c
.
20% of the marks for this assignment will come from manual marking of the readability of the C you have written. The manual marking will involve checking your code for clarity, and readability, which includes the use of functions and efficient use of loops and if statements.
Marks for your performance will be allocated roughly according to the below scheme.
100% for Performance | Completely Working Implementation, which exactly follows the specification (Stage 1, 2, 3 and 4). |
85% for Performance | Completely working implementation of Stage 1, 2 and 3. |
65% for Performance | Completely working implementation of Stage 1 and Stage 2. |
35% for Performance | Completely working implementation of Stage 1. |
Marks for your style will be allocated roughly according to the scheme below.
Style Marking Rubric
0 | 1 | 2 | 3 | 4 | |
Formatting (/5) | |||||
Indentation (/2) - Should use a consistent indentation scheme. | Multiple instances throughout code of inconsistent/bad indentation | Code is mostly correctly indented | Code is consistently indented throughout the program | ||
Whitespace (/1) - Should use consistent whitespace (for example, 3 + 3 not 3+ 3) | Many whitespace errors | No whitespace errors | |||
Vertical Whitespace (/1) - Should use consistent whitespace (for example, vertical whitespace between sections of code) | Code has no consideration for use of vertical whitespace | Code consistently uses reasonable vertical whitespace | |||
Line Length (/1) - Lines should be max. 80 characters long | Many lines over 80 characters | No lines over 80 characters | |||
Documentation (/5) | |||||
Comments (incl. header comment) (/3) - Comments have been used throughout the code above code sections and functions to explain their purpose. A header comment (with name, zID and a program description) has been included | No comments provided throughout code | Few comments provided throughout code | Comments are provided as needed, but some details or explanations may be missing causing the code to be difficult to follow | Comments have been used throughout the code above code sections and functions to explain their purpose. A header comment (with name, zID and a program description) has been included | |
Function/variable/constant naming (/2) - Functions/variables/constants names all follow naming conventions in style guide and help in understanding the code | Functions/variables/constants names do not follow naming conventions in style guide and help in understanding the code | Functions/variables/constants names somewhat follow naming conventions in style guide and help in understanding the code | Functions/variables/constants names all follow naming conventions in style guide and help in understanding the code | ||
Organisation (/5) | |||||
Function Usage (/4) - Code has been decomposed into appropriate functions separating functionalities | No functions are present, code is one main function | Some code has been moved to functions | Some code has been moved to sensible/thought out functions, and/or many functions exceed 50 lines (incl. main function) | Most code has been moved to sensible/thought out functions, and/or some functions exceed 50 lines (incl. main function) | All code has been meaningfully decomposed into functions of a maximum of 50 lines (incl. The main function) |
Function Prototypes (/1) - Function Prototypes have been used to declare functions above main | Functions are used but have not been prototyped | All functions have a prototype above the main function or no functions are used | |||
Elegance (/5) | |||||
Overdeep nesting (/2) - You should not have too many levels of nesting in your code (nesting which is 5 or more levels deep) | Many instances of overdeep nesting | <= 3 instances of overdeep nesting | No instances of overdeep nesting | ||
Code Repetition (/2) - Potential repetition of code has been dealt with via the use of functions or loops | Many instances of repeated code sections | <= 3 instances of repeated code sections | Potential repetition of code has been dealt with via the use of functions or loops | ||
Constant Usage (/1) - Any magic numbers are #defined | None of the constants used throughout program are #defined | All constants used are #defined and are used consistently in the code | |||
Illegal elements | |||||
Illegal elements - Presence of any illegal elements indicated in the style guide | CAP MARK AT 16/20 |
Note that the following penalties apply to your total mark for plagiarism:
0 for the assignment | Knowingly providing your work to anyone and it is subsequently submitted (by anyone). |
0 for the assignment | Submitting any other person's work. This includes joint work. |
0 FL for COMP1511 | Paying another person to complete work. Submitting another person's work without their consent. |
Allowed C Features
In this assignment, there are no restrictions on C Features, except for those in the style guide. If you choose to disregard this advice, you must still follow the style guide.
You also may be unable to get help from course staff if you use features not taught in COMP1511. Features that the Style Guide identifies as illegal will result in a penalty during marking. You can find the style marking rubric above. Please note that this assignment must be completed using only Linked Lists . Do not use arrays in this assignment. If you use arrays instead of lined lists you will receive a 0 for performance in this assignment.
Due Date
This assignment is due 26 April 2025 20:00:00. For each day after that time, the maximum mark it can achieve will be reduced by 5% (off the ceiling).- For instance, at 1 day past the due date, the maximum mark you can get is 95%.
- For instance, at 3 days past the due date, the maximum mark you can get is 85%.
- For instance, at 5 days past the due date, the maximum mark you can get is 75%.
Change Log
-
Version 1.0
(2025-04-03 09:00) -
- Assignment Released
-
Version 1.1
(2025-04-08 07:00) -
- Fix error handling in stage 2 for height requirements and if a visitor is already in the park.
-
Version 1.2
(2025-04-13 14:00) -
- Fix minor typos, bugs and formatting from forum posts in stages 2.4, 2.1 and 4.2.
-
Version 1.3
(2025-04-21 09:30) -
- Update Allowed C features to be more clear about what is permitted regarding arrays.
-
Version 1.4
(2025-04-22 13:30) -
- Update 4.1 reference implementation to be consistent with the specification.
-
Version 1.5
(2025-04-25 17:00) -
- Update 2.4 reference implementation error handling to be consistent with the specification. Note, these error messages will not be tested in marking.