version: 1.8 last updated: 2024-11-4 19:22
Assignment 2 - CS Dungeon!
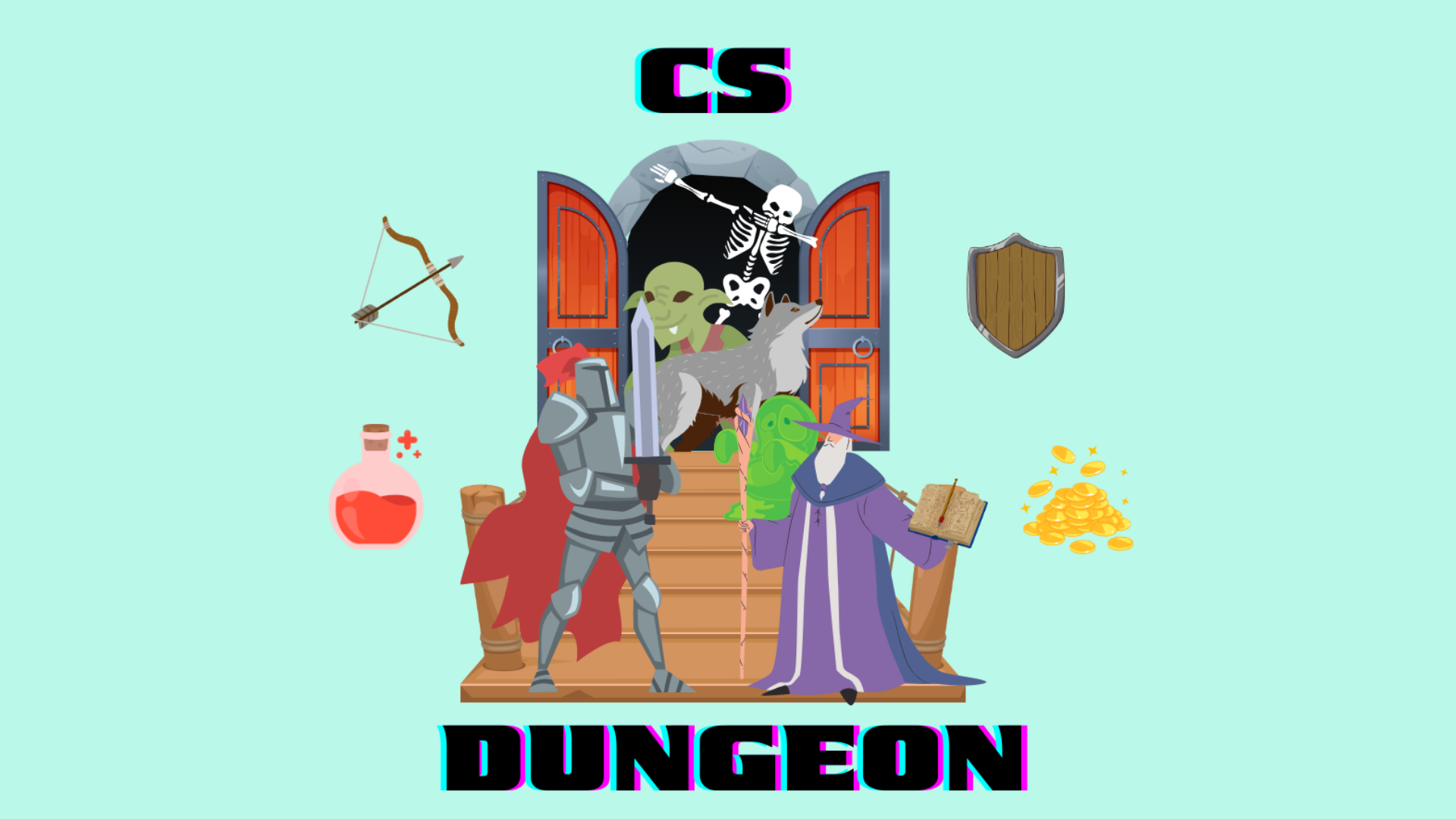
The game consists of a setup phase, where you add dungeons and items, and a gameplay phase, where you as the player will get to move between dungeons, fight monsters and collect items that can make you even stronger!
You can read about the history of dungeon crawler games here.
Overview
COMP1911 Students
If you are a COMP1911 student, you will be assessed on your performance in stages 1 and 2 ONLY for this assignment, which will make up the performance component of your grade. Style will be marked as usual and will make up the remaining 20% of the marks for this assignment. You are NOT required to attempt stages 3 and 4. You may attempt the later stages if you wish, but you will not be awarded any marks for work beyond stage 2.
COMP1511 Students
If you are a COMP1511 student, you will be assessed on your performance in stages 1 through 4, which will make up the performance component of your grade. Style will be marked as usual and will make up the remaining 20% of the marks for this assignment.
Assignment Structure
This assignment will test your ability to create, use, manipulate and solve problems using linked lists. To do this, you will be implementing a dungeon crawler game, where the dungeons are represented as a linked list, stored within a map. Each dungeon contains a list of items. The map also contains a player struct, which also contains a list of items.
We have defined some structs in the provided code to get you started. You may add fields to any of the structs if you wish.
struct map
-
Purpose: To store all the information about the dungeon map. It contains the list of dungeons within the map, the player, and the number of points required to win the game. The
entrance
field is a pointer to the first dungeon in the list of dungeons. -
Defined in
cs_dungeon.h
.
struct dungeon
-
Purpose: To store all the information about a single dungeon. This will form a linked list of dungeons by pointing to the next one (or
NULL
). -
Defined in
cs_dungeon.c
.
struct item
-
Purpose: To store all the information about a single item. This will form a linked list of items by pointing to the next one (or
NULL
). Items can be stored in a dungeon and in the player's inventory. -
Defined in
cs_dungeon.c
.
struct player
-
Purpose: To store all the information about the player.
-
Defined in
cs_dungeon.c
.
struct boss
-
Purpose: To store all the information about the final boss.
-
Defined in
cs_dungeon.c
.
The following enum definitions are also provided for you. You can create your own enums if you would like, but you should not modify the provided enums.
enum monster_type
-
Purpose: To represent the different types of monsters that can be encountered in the dungeons.
-
Defined in
cs_dungeon.h
.
enum item_type
-
Purpose: To represent the different types of items that can be found in the dungeons.
-
Defined in
cs_dungeon.h
.
Getting Started
There are a few steps to getting started with CS Dungeon.
- Create a new folder for your assignment work and move into it. You can follow the commands below to link and copy the files.
mkdir ass2 cd ass2
- There are 3 files in this assignment. Run the following command below to download all 3, which will link the header file and the main file. This means that if we make any changes to
cs_dungeon.h
ormain.c
, you will not have to download the latest version as yours will already be linked.
1511 fetch-activity cs_dungeon
- Run
1511 autotest cs_dungeon
to make sure you have correctly downloaded the file.
1511 autotest cs_dungeon
- Read through the rest of the introductory specification and Stage 1.
Starter Code
This assignment utilises a multi-file system. There are three files we use in CS Dungeon:
-
Main File (
main.c
): This file handles all input scanning and error handling for you. It also tests your code incs_dungeon.c
and contains themain
function. You don't need to modify or fully understand this file, but if you're curious about how this assignment works, feel free to take a look. You cannot changemain.c
. -
Header File (
cs_dungeon.h
): contains defined constants that you can use and function prototypes. It also contains header comments that explain what functions should do and their inputs and outputs. If you are confused about what a function should do, read the header file and the corresponding specification. You cannot changecs_dungeon.h
. -
Implementation File (
cs_dungeon.c
): contains stubs of functions for you to implement. This file does not contain amain
function, so you will need to compile it alongsidemain.c
. This is the only file you may change. You do not need to usescanf
orfgets
anywhere.
The implementation file cs_dungeon.c
contains some provided functions to help simplify some stages of this assignment. These functions have been fully implemented for you and should not need to be modified to complete this assignment.
These provided functions will be explained in the relevant stages of this assignment. Please read the function comments and the specification as we will suggest certain provided functions for you to use.
How to Compile CS Dungeon
To compile you should compile cs_dungeon.c
alongside main.c
. This will allow you to run the program yourself and test the functions you have written in cs_dungeon.c
. Autotests have been written to compile your cs_dungeon.c
with the provided main.c
.
To compile your code, use the following command:
dcc cs_dungeon.c main.c -o cs_dungeon
Once your code is compiled, you can run it with the following command:
./cs_dungeon
To autotest your code, use the following command:
1511 autotest cs_dungeon
Reference Implementation
To help you understand the expected behaviour of CS Dungeon, we have provided a reference implementation. If you have any questions about the behaviour of your assignment, you can check and compare yours to the reference implementation.
To run the reference implementation, use the following command:
1511 cs_dungeon
Once you have followed the setup prompts, you might want to start by running the ?
command, whether you are in the setup phase or the gameplay phase.
When in the setup phase, the ?
command will display what you can add to the map:
1511 cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: ? =======================[ 1511 Dungeon ]======================= =============[ Setup: Usage Info ]=============== ? Show setup usage info q Exit setup stage a [dungeon name] [monster type] [num_monsters] Append a dungeon to the end of the map's list of dungeons p Prints the map's list of dungeons s Shows the player's current stats i [position] [dungeon name] [monster type] [num_monsters] Inserts a dungeon to the specified position in the map t [dungeon position] [item type] [points] Inserts an item into the specified dungeon's list of items ================================================================ Enter Command: No dungeons have been added, so it's not possible to play the game! Exiting.
When in the gameplay phase, the ?
command will show you what actions the player can take:
1511 cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a beach 1 1 beach has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 1 The final boss has been added to faerun! Map of faerun! |^|^|^|^|^| |^|^|^|^|^| 1. beach Boss: Present Monster: Slime Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command:? =======================[ 1511 Dungeon ]======================= =============[ Gameplay: Usage Info ]=============== ? Show gameplay usage info q Exit gameplay p Prints the map's list of dungeons s Shows the player's current stats d Prints the current dungeon's details > Move to the next dungeon in the map < Move to the previous dungeon in the map ! Physically attack monsters in current dungeon # Magically attack monsters in current dungeon P Activate the player's class power c [item number] Collect the specified item from current dungeon u [item number] Use the specified item from the player's inventory T Teleport to the furthest dungeon b Fight the boss in the current dungeon ================================================================ Enter Command: Thanks for playing!
The easiest way to understand how this assignment works is to play a game yourself! Below is example input you can try by using the reference solution.
Faerun 25 Minsc Fighter a cavern 1 3 a castle 2 2 a graveyard 3 3 t 1 0 5 t 1 0 3 t 2 1 5 t 2 3 5 t 2 3 5 q 1
Allowed C Features
In this assignment, there are no restrictions on C Features, except for those in the Style Guide. If you choose to disregard this advice, you must still follow the Style Guide.
You also may be unable to get help from course staff if you use features not taught in COMP1511. Features that the Style Guide identifies as illegal will result in a penalty during marking. You can find the style marking rubric above. Please note that this assignment must be completed using only Linked Lists . Do not use arrays in this assignment. If you use arrays instead of linked lists you will receive a 0 for performance in this assignment.
Banned C Features
In this assignment, you cannot use arrays for the list of dungeons nor the lists of items and cannot use the features explicitly banned in the Style Guide. If you use arrays in this assignment for the linked list of dungeons or the linked lists of items you will receive a 0 for performance in this assignment.
FAQ
Q: Can I edit the given starter code functions/structs/enums?
You can only edit cs_dungeon.c
.You cannot edit anything in main.c
or cs_dungeon.h
.
You cannot edit any of the parameters or names for the provided functions.
Q: Can I use X other C feature
A: For everything not taught in the course, check the style guide. If it says "Avoid", then we may take style marks off if its not used correctly. If it says "Don't Use" then we will take style marks off (see the style marking rubric).
Game Structure
This game consists of a setup phase and a gameplay phase.
You will be implementing both the setup phase and gameplay phase throughout this assignment, adding more features to both as you progress. By the end of **stage 1.4****you will have implemented parts of both setup and gameplay enough to play a very basic game.
The game is ended either by the user entering Ctrl-D
, the win condition being met, or the player running out of health points. The program can also be ended in the setup phase with Ctrl-D
or q
.
Your Tasks
This assignment consists of four stages. Each stage builds on the work of the previous stage, and each stage has a higher complexity than its predecessor. You should complete the stages in order.
A video explanation to help you get started with the assignment can here found here:
Stage 1
For Stage 1 of this assignment, you will be implementing some basic commands to set up your dungeon map and play a simple game!
Stage 1.1 Creating the Map, a Dungeon, and the Player
In Stage 1.1, you'll implement functions that allocate memory using malloc
and initialise all fields for struct map
, struct dungeon
, and struct player
.
You will find the following unimplemented function stubs in cs_dungeon.c
:
struct map *create_map(char *name, int win_requirement) {
// TODO: implement this function
printf("Create Map not yet implemented.\n");
exit(1);
}
struct dungeon *create_dungeon(char *name,
enum monster_type monster,
int num_monsters,
int contains_player) {
// TODO: implement this function
printf("Create Dungeon not yet implemented.\n");
exit(1);
}
struct player *create_player(char *name, char *class_type) {
// TODO: implement this function
printf("Create Player not yet implemented.\n");
exit(1);
}
In main.c
, create_map
is called after reading the map name and amount of points required to win, and create_player
is called after reading the player name and class. create_dungeon
will be called by you, in cs_dungeon.c
, whenever a dungeon should be added to the map.
Your task is to implement the create_map
function, so that it:
- Creates a new
struct map
(usingmalloc
). - Copies the
name
andwin_requirement
arguments into the corresponding struct fields. - Initialises all other fields to a reasonable value.
- Returns a pointer to the newly created
struct map
.
You also then need to complete the create_player
function, so that it:
- Creates a new
struct player
(usingmalloc
). - Copies the
name
andclass_type
arguments into the corresponding struct fields. - Initialises
health_points
,shield_power
,damage
andmagic_modifier
based on the chosen class (listed below). - Initialises all other fields to some reasonable value.
- Returns a pointer to the newly created
struct player
.
Class | Fighter | Wizard |
---|---|---|
Health Points | 30 | 15 |
Shield Power | 2 | 0 |
Damage | 8 | 7 |
Magic Modifier | 0.9 | 1.5 |
You also then need to complete the create_dungeon
function, so that it:
- Creates a new
struct dungeon
(usingmalloc
). - Copies the
name
,monster
,num_monsters
andcontains_player
arguments into the corresponding struct fields. - Initialises all other fields to some reasonable value.
- Returns a pointer to the newly created
struct dungeon
.
Clarifications
- Initially there are no dungeons in the map.
- Initially there are no items in a dungeon, and no items in the player's inventory.
- No error handling is required for Stage 1.1.
Testing
There are no autotests for Stage 1.1.
Instead, you may want to double check your work by compiling your code using dcc
and making sure there are no warnings or errors. Make sure that every struct field is initialised. If there are any errors in your code, they will become clear in Stage 1.2 onwards.
You can optionally use the file cs_dungeon_stage1_testing.c
to test your functions. This file should not be submitted, it is only for basic testing, to ensure all your struct fields have been initialised.
This file is not used in testing when marking your submissions, do not submit it.
To fetch this file, use the following command:
1511 fetch-testing cs_dungeon_stage1_testing
This testing file contains the line #include "cs_dungeon."
, so that we can call your functions (create_map
, create_player
and create_dungeon
) from cs_dungeon.c
in this file. This means you do not need to edit this file (but can if you wish to)! Once you are happy with your code for these functions, you can test your implementation like below:
dcc cs_dungeon_stage1_testing.c cs_dungeon.c -o cs_dungeon_stage1_testing ./cs_dungeon_stage1_testing Map name: Faerun Win requirement: 25 Player is NULL Entrance is NULL Player name: Minsc Class type: Fighter Damage: 8 Health points: 30 Shield power: 2 Magic modifier: 0.900000 Points: 0 Inventory is NULL Dungeon name: forest Number of monsters: 2 Contains player: 1 Dungeon contains skeletons. Items is NULL Boss is NULL Next is NULL
Stage 1.2 Appending Dungeons
Now it’s time to start building out your dungeon map! When you run your program, create_map
and create_player
will be called for you by main.c
. Then, the setup phase loop will start, where you can begin to create your own custom dungeon map!
The first command you will be implementing is the Append Dungeon command.
Command: Append Dungeon
Enter Command: a [name] [monster] [num_monsters]
Before appending any dungeons, the map's list of dungeons will be empty, looking something like this:
After appending one dungeon, there will be one dungeon in the map, so that it looks something like this:
Any other dungeons added via this command will all be appended to the end of the list, i.e. a tail insertion, looking something like this:
Your task is to implement the function append_dungeon
in cs_dungeon.c
which will append a newly created dungeon to the end of the map's list of dungeons, where entrance
is the first dungeon in the list.
Additionally, the first dungeon in the map, i.e. what entrance
points to, is where the player should start, so it should contain the player. If a dungeon contains the player, the contains_player
field should be set to 1
. Otherwise, it should be set to 0
.
You will need to:
- Create a new dungeon using your
create_dungeon
function from Stage 1.1, using the parameters provided. - Append the new dungeon to the end of the list of dungeons stored in the map, which starts at the
entrance
(head of the list), i.e. perform a tail insertion.
This function returns an int
- this value indicates whether or not the appending was successful or not. We will handle error checking in Stage 1.5, so for now you can return the constant VALID
(defined in cs_dungeon.h
). main.c
will handle all input and output (including any printf
, scanf
, or fgets
calls) for you already.
Clarifications
- The first dungeon in the map is where the player should begin.
- The
boss
field can be left asNULL
. The final boss will be added in Stage 1.5. - No error handling is required for Stage 1.2
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a sewer 1 3 sewer has been added as a dungeon to the map! Enter Command: Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: UNSW Please enter the amount of points required to win: 40 Please enter the player's name: Bob Player Class Options: Fighter Wizard Please enter player's chosen class type: Wizard ----------Setup Phase---------- Enter Command: a classroom 1 1 classroom has been added as a dungeon to the map! Enter Command: a forest 2 2 forest has been added as a dungeon to the map! Enter Command: a lab 3 3 lab has been added as a dungeon to the map! Enter Command: Thanks for playing!
Stage 1.3 - Printing the Dungeon Map
Now we want to be able to display all dungeons in the map and their basic details.
Command: Print dungeons
Enter Command: p
When the p
command is run, your program should print out all of the dungeons stored in the map and their basic information. This is each dungeon’s position (in order of head to tail, indexed from 1), name, if the boss is present, and if the player is currently in that dungeon. This command can be called in both the setup phase and the gameplay phase, main.c
will handle this for you.
There is one function stub to implement for Stage 1.3, called print_map
in cs_dungeon.c
.
Four functions have been provided for you in cs_dungeon.c
to help print the list of dungeons:
print_empty_map
: prints an empty map message.print_map_name
: prints a message saying "Map of [name]".print_basic_dungeon
: prints the basic details of a given dungeon.print_connection
: prints a tunnel connecting adjacent dungeons.
You will need to:
- Print every dungeon in the list stored in the map, using the provided printing functions.
Clarifications
- The map should only be empty in the setup phase, before any dungeons have been added.
- No error handling is required for Stage 1.3
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: UNSW Please enter the amount of points required to win: 30 Please enter the player's name: Bob Player Class Options: Fighter Wizard Please enter player's chosen class type: Wizard ----------Setup Phase---------- Enter Command: a classroom 3 3 classroom has been added as a dungeon to the map! Enter Command: p Map of UNSW! |^|^|^|^|^| |^|^|^|^|^| 1. classroom Boss: None Monster: Skeleton Bob is here |^|^|^|^|^| |^|^|^|^|^| Enter Command: Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a Sewers 1 3 Sewers has been added as a dungeon to the map! Enter Command: a Desert 2 2 Desert has been added as a dungeon to the map! Enter Command: a Forest 3 4 Forest has been added as a dungeon to the map! Enter Command: a Town 4 1 Town has been added as a dungeon to the map! Enter Command: p Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. Sewers Boss: None Monster: Slime Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. Desert Boss: None Monster: Goblin Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 3. Forest Boss: None Monster: Skeleton Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 4. Town Boss: None Monster: Wolf Empty |^|^|^|^|^| |^|^|^|^|^| Enter Command: Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: UNSW Please enter the amount of points required to win: 30 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: p There are no dungeons currently in the dungeon. Enter Command: Thanks for playing!
Stage 1.4 Adding the Final Boss
So that we can move on to the gameplay component of CS Dungeon, we need to add a boss-level monster to the final dungeon in the map.
When q
command is used in the setup phase, the player will then be prompted to enter the item type required to defeat the final boss:
Please enter the required item to defeat the final boss: [item type]
Then, the function final_boss
in cs_dungeon.c
will be called, which you will need to implement in this stage. This function returns an int
- this value indicates whether or not adding the final boss was successful or not. We will handle error checking in Stage 1.5, so for now you can return the constant VALID
(defined in cs_dungeon.h
).
The map should always have at least one dungeon in it when it is time to add the final boss, and throughout the gameplay phase (the one the player is in).
The final boss's stats are as follows:
Health Points | 35 |
---|---|
Damage | 10 |
Points | 20 |
There are two functions to implement in Stage 1.4, create_boss
and final_boss
. You will need to:
- Create a
struct boss
(usingmalloc
) similarly to the create functions in Stage 1.1 in thecreate_boss
function. - Add this final boss to the very last dungeon in the map, specifically to the dungeon's
boss
field in thefinal_boss
function.
After you have completed this stage, you will be able to play a very basic game of CS Dungeon, where you can add dungeons, a final boss, and print the map!
Clarifications
- There will always be at least one dungeon in the before moving to the gameplay phase (
main.c
checks for this). - No error handling is required for Stage 1.4.
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a Sewers 1 3 Sewers has been added as a dungeon to the map! Enter Command: a Desert 2 2 Desert has been added as a dungeon to the map! Enter Command: a Forest 3 4 Forest has been added as a dungeon to the map! Enter Command: a Town 4 1 Town has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 0 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. Sewers Boss: None Monster: Slime Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. Desert Boss: None Monster: Goblin Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 3. Forest Boss: None Monster: Skeleton Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 4. Town Boss: Present Monster: Wolf Empty |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: Thanks for playing!
Stage 1.5 Handling Errors
Now we need to make sure that when setting up our map, that any invalid dungeon or boss inputs are handled correctly. From Stage 1.5 onwards, you will need to do error checking to ensure correct inputs when creating a dungeon and will need to edit your code from earlier stages.
You will need to check for the following errors in your append_dungeon
and final_boss
functions:
- Invalid name,
- Invalid monster type,
- Invalid monster amount,
- Invalid required item,
and if they are, return the corresponding constant. You’re currently returning VALID
by default.
The error constants are defined in cs_dungeon.h
, and you can refer to the header comments for append_dungeon
and final_boss
for which constants to return in each case.
To check whether each field is valid or not, you must perform the following checks in the below order:
Appending dungeons
name
is invalid if it is already being used by another dungeon in the map.monster
is invalid if it is not one ofSLIME
,GOBLIN
,SKELETON
orWOLF
.num_monsters
is invalid if it is not between 1 and 10 (inclusive).
Creating the final boss
required_item
is invalid if it is not one ofPHYSICAL_WEAPON
,MAGICAL_TOME
,ARMOR
,HEALTH_POTION
orTREASURE
.
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a sewer 10 5 ERROR: Invalid monster type. Monster type should be one of: 1: SLIME 2: GOBLIN 3: SKELETON 4: WOLF Enter Command: a sewer 1 100 ERROR: Invalid monster number. Monster number must be between 1 and 10. Enter Command: a sewer 1 3 sewer has been added as a dungeon to the map! Enter Command: a sewer 3 1 ERROR: Invalid name. This name already exists in the map. Enter Command: a beach 100 100 ERROR: Invalid monster type. Monster type should be one of: 1: SLIME 2: GOBLIN 3: SKELETON 4: WOLF Enter Command: q Please enter the required item to defeat the final boss: -10 ERROR: Invalid item type. Item type should be one of: 0: PHYSICAL WEAPON 1: MAGICAL TOME 2: ARMOR 3: HEALTH POTION 4: TREASURE Please enter the required item to defeat the final boss: 1 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. sewer Boss: Present Monster: Slime Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: Thanks for playing!
Stage 1.6 - Checking Player Stats
We need a way to check what the player’s statistics are while we are playing our game. So in Stage 1.6 you will be implementing a feature that checks the player's current stats, specifically:
- what dungeon they are in,
- their health points,
- shield power,
- damage,
- magic modifier,
- points collected,
- and what items they have in their inventory.
Command: Check Player Stats
Enter Command: s
There is one function to implement for Stage 1.6, called player_stats
in cs_dungeon.c
.
There are 3 helper functions provided for you in cs_dungeon.c
to help you print everything:
print_player
: prints the details about the player.print_no_items
: if there are no items in the player's inventory, this should be printed.print_item
this prints the details of a given item. This does not need to be used until Stage 3, when items are added.
You will need to:
- Find the above listed information about the player.
- Use the provided printing functions to print the player's stats.
Clarifications
- If the map is empty e.g. in the setup phase before adding any dungeons, then
NULL
should be given as the dungeon name toprint_player
. - Items are not added until Stage 3, so you do not need to use
print_item
until then. Instead, useprint_no_items
for now. - There is no error handling in Stage 1.6.
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a Sewers 1 3 Sewers has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 0 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. Sewers Boss: Present Monster: Slime Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: s =======Player Stats======= Minsc is currently in Sewers Fighter Health Points: 30 Shield Power: 2 Damage: 8 Magic Modifier: 0.9 Points Collected: 0 Minsc has the following items in their inventory: No Items Enter Command: Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: UNSW Please enter the amount of points required to win: 30 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: s =======Player Stats======= Minsc has not entered the map yet! Fighter Health Points: 30 Shield Power: 2 Damage: 8 Magic Modifier: 0.9 Points Collected: 0 Minsc has the following items in their inventory: No Items Enter Command: Thanks for playing!
Testing and Submission
Remember to do your own testing
Are you finished with this stage? If so, you should make sure to do the following:
- Run
1511 style
and clean up any issues a human may have reading your code. Don't forget -- 20% of your mark in the assignment is based on style and readability! - Autotest for this stage of the assignment by running the
autotest-stage
command as shown below. - Remember -- give early and give often. Only your last submission counts, but why not be safe and submit right now?
1511 style cs_dungeon.c 1511 autotest-stage 01 cs_dungeon give cs1511 ass2_cs_dungeon cs_dungeon.c
Stage 2
In Stage 2 of this assignment, you will be using more advanced linked list concepts to add and move nodes within a linked list and perform operations using the values stored inside those nodes.
Specifically, this will include:
- Inserting a dungeon into the map at a specified position.
- Print details about the dungeon the player is currently in.
- Move the player between dungeons.
- Fight monsters (not the final boss).
- Implement class-specific powers.
Stage 2.1 - Inserting Dungeons
Currently, we have a way of appending a dungeon to the end of the map's list of dungeons, but there's no way to insert a dungeon anywhere else in your list.
That's why you will be implementing the Insert Dungeon command for Stage 2.1, which will insert a new dungeon at the specified position, rather than at the end of the map's list of.
Enter Command: i [position] [name] [monster] [num_monsters]
Inserting a new dungeon should look something like this:
Before Inserting:
After Inserting:
For this stage, you will need to implement the function insert_dungeon
in cs_dungeon.c
.
For both of these functions, your program should create a new struct dungeon
and then insert it into the map's list of dungeons at the given position
. The list is indexed from 1, meaning the head of the list is position 1. The position
given means that the new dungeon should take that position in the list, e.g. if the position was 1
, the new dungeon should become the new entrance
of the map's list of dungeons.
This function returns an int
- this value indicates whether or not the inserting was successful or not. As we handled error checking in Stage 1.5, you will now need to check if any of the arguments provided are invalid. If they are, you will need to return the appropriate constant, defined in cs_dungeon.h
.
main.c
will then handle the printing accordingly.
If a dungeon is invalid, it should not be inserted to the list of dungeons in the map.
Clarifications
- Your program should also check if
position
is invalid.position
is invalid if it is less than 1. - Your program should handle the same errors as stage 1.5. Invalid position should be checked first, then the rest of the errors in the same order as Stage 1.5.
- The list is indexed from 1, meaning the head of the list (
entrance
) is position 1. Theposition
given means that the new dungeon should take that position in the list, and should point to the dungeon that previously held that position. - If the given
position
is 1, the new dungeon should become the new entrance of the map. - As stated in Stage 1.2, the player should start in the
entrance
dungeon of the map, so you will need to update this as well. - If the given
position
is larger than the length of the list, the new dungeon should be added to the tail of the list.
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: i 1 forest 3 2 forest has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 1 Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: Present Monster: Skeleton Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a beach 1 2 beach has been added as a dungeon to the map! Enter Command: a castle 2 2 castle has been added as a dungeon to the map! Enter Command: i 3 forest 3 2 forest has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 1 The final boss has been added to Fearun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. beach Boss: None Monster: Slime Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. castle Boss: None Monster: Goblin Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 3. forest Boss: Present Monster: Skeleton Empty |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a beach 1 2 beach has been added as a dungeon to the map! castle Command: a castle 2 2 castle has been added as a dungeon to the map! Enter Command: i 2 forest 3 2 forest has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 1 Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. beach Boss: None Monster: Slime Misc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. forest Boss: None Monster: Skeleton Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 3. castle Boss: Present Monster: Goblin Empty |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: i 0 forest 3 2 ERROR: Invalid position. Position must be 1 or greater. Enter Command: Thanks for playing!
Stage 2.2 - Print Current Dungeon Details
We need a way to see all the details about the dungeon the player is currently in, specifically:
- How many monsters are in the dungeon
- Boss details (if there is a boss)
- All items in the dungeon (at this stage there should be no items)
Enter Command: d
There is one function in cs_dungeon.c
that you will have to implement for Stage 2.2, print_dungeon
.
Another helper function has been provided to you, in addition to print_no_items
and print_item
from Stage 1.6. You do not need to use print_item
until items are added in Stage 3.
print_detail_dungeon
prints all the specific details about a dungeon, as listed above.
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a forest 3 2 forest has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 0 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: Present Monster: Skeleton Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: d ======Dungeon Details====== Minsc is currently in forest There are 2 Skeletons The boss is in this dungeon Health Points: 35 Damage: 10 Points: 20 Required Item: Physical Weapon The dungeon forest has the following items: No Items Enter Command: Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a forest 3 2 forest has been added as a dungeon to the map! Enter Command: a cave 1 1 cave has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 0 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: None Monster: Skeleton Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. cave Boss: Present Monster: Slime Empty |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: d ======Dungeon Details====== Minsc is currently in forest There are 2 Skeletons No boss in this dungeon The dungeon forest has the following items: No Items Enter Command: Thanks for playing!
Stage 2.3 - Move Player Between Dungeons
We’ve created a map with a list of dungeons, now we want to be able to actually move the player through the dungeons!
Moving forward to the next dungeon:
Enter Command: >
Moving backward to the previous dungeon:
Enter Command: <
There is one function in cs_dungeon.c
that you will have to implement for Stage 2.3. move_player
, which will move the player from the dungeon they are currently in, to an adjacent dungeon, depending on whether >
or <
was inputted.
This function returns an int
- this value indicates whether or not the moving was successful or not. Return VALID
if there is a dungeon to move into, and INVALID
if not.
main.c
will then handle the printing accordingly.
Clarifications
- If there is no dungeon the player can move into, the player should not move.
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a forest 3 2 forest has been added as a dungeon to the map! Enter Command: a cave 1 1 cave has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 0 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: None Monster: Skeleton Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. cave Boss: Present Monster: Slime Empty |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: > Moved into the next dungeon Enter Command: p Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: None Monster: Skeleton Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. cave Boss: Present Monster: Slime Minsc is here |^|^|^|^|^| |^|^|^|^|^| Enter Command: < Moved into the previous dungeon Enter Command: p |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: None Monster: Skeleton Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. cave Boss: Present Monster: Slime Empty |^|^|^|^|^| |^|^|^|^|^| Enter Command: < ERROR: Invalid move. There must be a dungeon next to the player to move into. Enter Command: p Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: None Monster: Skeleton Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. cave Boss: Present Monster: Slime Empty |^|^|^|^|^| |^|^|^|^|^| Enter Command: Thanks for playing!
Stage 2.4 - Fight Monsters
Now we get to really get into our dungeon crawler game, let’s fight some monsters! There are two ways to fight monsters, with physical attacks, or magical attacks. These attacks do not apply to boss-level monsters. So in Stage 2.4, you will need to implement these commands.
Physical attacks (damage
field in struct player
):
Enter Command: !
Magical attacks (damage
multiplied by magic_modifier
in struct player
):
Enter Command: #
Rules: Short Version/Summary
- Monsters have health and attack damage based on their enum value, but the player's shield reduces some of the damage (based on shield power).
- The player can attack all monsters in the current dungeon with either a physical attack (
damage
) or a magical attack (damage
multiplied bymagic modifier
). - If any monsters survive the attack, they 'heal' and will be at full health for the next turn.
- Once monsters have been attacked, monsters in that dungeon will continue to attack the player each turn. Wolves, however, attack the player every turn, regardless of what happens.
Fighter with 4 damage fights 2 goblins:
Fighter with 3 damage fights 4 goblins:
- Both a monster's health and damage is indicated by their enum value, e.g. slimes have 1 health point and do 1 damage.
- In a given dungeon,
monster
represents what type of monster is within, andnum_monsters
represents how many of that monster there are. - When the player attacks, they can defeat as many monsters as they have damage to defeat. e.g. if the player had 4 damage, they could defeat 2 goblins (enum value of 2).
- If a player does not have enough damage to completely defeat a monster, the monster is not defeated. e.g. if the player had 3 damage, they could only defeat 1 goblin (enum value of 2).
- Any monster that was not defeated keeps their original health (so there is no monster health tracking between turns). e.g. if there were 3 goblins, and the player did 5 damage, there would still be one goblin left. Thematically, the monsters rest and heal between turns.
- The player should collect points after a battle totalling the number of monsters defeated multiplied by the monster's enum value.
- After being attacked for the first time, monsters will now fight the player at the end of each turn (when they are in the same dungeon), dealing damage equal to their enum value multiplied by the amount of monsters left. e.g. if there was 1 goblin left in a dungeon after fighting, at the end of each turn the goblin would deal 2 damage to the player (when they are in the same dungeon).
- If the player has any shielding, the damage from the monster is decreased by the amount of shielding the player has. e.g. if the player had 1 shielding, a goblin's damage would be 1.
There are two functions in cs_dungeon.c
that you will have to implement for Stage 2.4, fight
and end_turn
. fight
will handle the fight between the player and the monster in the current turn, and end_turn
will handle any extra actions that will need to occur at the end of each turn, e.g. attacked monsters and wolves. This will be built upon further in later stages.
fight
is called when a physical attack or magical attack command is inputted. end_turn
is called by main.c
at the end of each turn, after a command has been executed.
fight
returns an int
- this value indicates whether or not the fight was successful or not. Return INVALID
if there are no monsters to attack, and VALID
otherwise.
end_turn
returns an int
- this value indicates whether or not the game is over. Return CONTINUE_GAME
for now, ending the game will be handled in Stage 3.1.
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a forest 3 3 forest has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 0 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: Present Monster: Skeleton Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: d ======Dungeon Details====== Minsc is currently in forest There are 3 Skeletons The boss is in this dungeon Health Points: 35 Damage: 10 Points: 20 Required Item: Physical Weapon The dungeon forest has the following items: No Items Enter Command: ! A battle has raged! Enter Command: d ======Dungeon Details====== Minsc is currently in forest There are 1 Skeletons The boss is in this dungeon Health Points: 35 Damage: 10 Points: 20 Required Item: Physical Weapon The dungeon forest has the following items: No Items Enter Command: ! A battle has raged! Enter Command: d ======Dungeon Details====== Minsc is currently in forest There are 0 Skeletons The boss is in this dungeon Health Points: 35 Damage: 10 Points: 20 Required Item: Physical Weapon The dungeon forest has the following items: No Items Enter Command: Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a forest 3 4 forest has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 0 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: Present Monster: Skeleton Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: d ======Dungeon Details====== Minsc is currently in forest There are 4 Skeletons The boss is in this dungeon Health Points: 35 Damage: 10 Points: 20 Required Item: Physical Weapon The dungeon forest has the following items: No Items Enter Command: s =======Player Stats======= Minsc is currently in forest Fighter Health Points: 30 Shield Power: 2 Damage: 8 Magic Modifier: 0.9 Points Collected: 0 Minsc has the following items in their inventory: No Items Enter Command: ! A battle has raged! Enter Command: d ======Dungeon Details====== Minsc is currently in forest There are 2 Skeletons The boss is in this dungeon Health Points: 35 Damage: 10 Points: 20 Required Item: Physical Weapon The dungeon forest has the following items: No Items Enter Command: s =======Player Stats======= Minsc is currently in forest Fighter Health Points: 22 Shield Power: 2 Damage: 8 Magic Modifier: 0.9 Points Collected: 6 Minsc has the following items in their inventory: No Items Enter Command: Thanks for playing!
Clarifications
- Shields do not decay.
- Wolves will fight the player without needing to be attacked first. This will need to be implemented in the
end_turn
function. - Monsters attack after the player, so if the player defeats any monsters in their turn, those defeated monsters cannot attack the player (as they have been defeated).
- Monsters that have been attacked by the player will continue to attack when the player leaves and returns to their dungeon, their change of state persists.
- Only monsters that have been attacked will attack the player, monsters in other dungeons remain dormant and will not attack the player until the player attacks them specifically, except for the
WOLF
type monster. - Monsters only do damage to the player in the
end_turn
function, which is called for you inmain.c
at the end of each turn. - Any command entered counts as a turn (even player stats(
s
), display current dungeon details (d
) and print map (p
)), so monsters can attack on these turns (if they have already been attacked once by the player). - Shielding applies to the total damage dealt by all monsters attacking, not each individual monster.
Stage 2.5 - Class Powers
Now we can unleash the special powers of the player's chosen class!
Enter Command: P
When the P
command is entered, the player’s class ability is activated. This can only be used once per game.
If the player is a Fighter, their damage is now permanently increased, 1.5 times.
If the player is a Wizard, they defeat all monsters in the current dungeon, except for the final boss.
If the special power has already been used this game, an error message should be printed and nothing should happen.
There is one function in cs_dungeon.c
that you will have to implement for Stage 2.5, class_power
.
This function returns an int
- this value indicates whether or not using the class power was successful. Return VALID
if the power was used, and INVALID
if not.
Clarifications
- When a Wizard defeats all monsters in the current dungeon, they also collect all the points those monsters were worth.
- A power can only be used if it has not already been used this game (i.e. class powers can only be used once per game).
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a forest 3 2 forest has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 0 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: Present Monster: Skeleton Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: s Minsc is currently in forest Fighter Health Points: 30 Shield Power: 2 Damage: 8 Magic Modifier: 0.9 Points Collected: 0 Minsc has the following items in their inventory: No Items Enter Command: P Minsc used their class power! This can no longer be used. Enter Command: s Minsc is currently in forest Fighter Health Points: 30 Shield Power: 2 Damage: 12 Magic Modifier: 0.9 Points Collected: 0 Minsc has the following items in their inventory: No Items Enter Command: Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Wizard ----------Setup Phase---------- Enter Command: a forest 3 2 forest has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 0 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: Present Monster: Skeleton Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: s ======Dungeon Details====== Minsc is currently in forest Wizard Health Points: 15 Shield Power: 0 Damage: 7 Magic Modifier: 1.5 Points Collected: 0 Minsc has the following items in their inventory: No Items Enter Command: d ======Dungeon Details====== Minsc is currently in forest There are 2 Skeletons The boss is in this dungeon Health Points: 35 Damage: 10 Points: 20 Required Item: Physical Weapon The dungeon forest has the following items: No Items Enter Command: P Minsc used their class power! This can no longer be used. Enter Command: d ======Dungeon Details====== Minsc is currently in forest There are 0 Skeletons The boss is in this dungeon Health Points: 35 Damage: 10 Points: 20 Required Item: Physical Weapon The dungeon forest has the following items: No Items Enter Command: s ======Dungeon Details====== Minsc is currently in forest Wizard Health Points: 15 Shield Power: 0 Damage: 7 Magic Modifier: 1.5 Points Collected: 6 Minsc has the following items in their inventory: No Items Enter Command: Thanks for playing!
Testing and Submission
Remember to do your own testing
Are you finished with this stage? If so, you should make sure to do the following:
- Run
1511 style
and clean up any issues a human may have reading your code. Don't forget -- 20% of your mark in the assignment is based on style and readability! - Autotest for this stage of the assignment by running the
autotest-stage
command as shown below. - Remember -- give early and give often. Only your last submission counts, but why not be safe and submit right now?
1511 style cs_dungeon.c 1511 autotest-stage 02 cs_dungeon give cs1511 ass2_cs_dungeon cs_dungeon.c
COMP1911 Students
Stage 3
In Stage 3 of this assignment, you will be manipulating 2D linked lists by adding lists of items to the dungeons and to the player. You will also be managing your memory usage by freeing memory and preventing memory leaks.
Specifically, this will include:
- Ending the game.
- Adding items to dungeons in the setup phase.
- Collecting items in dungeons.
- Using items.
- Removing empty dungeons.
Stage 3.1 - Ending the game
Finally, we can end our game! Once the player has collected the point requirement (stored in map->win_requirement
) and has either defeated the final boss (handled in Stage 4), or defeated all other monsters, the player has won the game! If the player has run out of health points, they lose the game.
To win the game, players must collect the required points and either defeat all monsters or take down the boss-level monster.
The game is lost if the player's health drops to 0 or below.
To implement this, you will need to add more functionality to your end_turn
function, as after each player turn, you should check to see if the game is over. At this point in the assignment, a turn is as follows:
- player action
- monster attacks
- check if the game is over
In end_turn
, you should return PLAYER_DEFEATED
if their health points drops to 0 or less. You should return WON_MONSTERS
if the player has defeated all monsters in the map and met the point requirement. You should return WON_BOSS
if the player has defeated the boss and met the point requirement (fighting the boss will be handled in Stage 4.2).
Clarifications
- If the player both meets the win criteria (collecting the required points and defeating either all monsters or the boss monster) and runs out of health points in the same turn, the player loses the game (you can't win if you've been defeated - you need to be able to leave the dungeon!).
- Testing for this stage will only use maps with one dungeon.
- There may be some games that are impossible to win, however, you do not need to account for these cases.
- The game can still be ended by inputting
CTRL + D
, with no need to check if the player has won or lost. - The boss-level monster is defeated when their health points reach 0.
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 9 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Wizard ----------Setup Phase---------- Enter Command: a forest 3 3 forest has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 1 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: Present Monster: Skeleton Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: # A battle has raged! Minsc the Wizard has won by collecting 9 points and defeating all monsters! Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 9 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Wizard ----------Setup Phase---------- Enter Command: a forest 4 2 forest has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 1 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: Present Monster: Wolf Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: s =======Player Stats======= Minsc is currently in forest Wizard Health Points: 15 Shield Power: 0 Damage: 7 Magic Modifier: 1.5 Points Collected: 0 Minsc has the following items in their inventory: No Items Enter Command: s =======Player Stats======= Minsc is currently in forest Wizard Health Points: 7 Shield Power: 0 Damage: 7 Magic Modifier: 1.5 Points Collected: 0 Minsc has the following items in their inventory: No Items Minsc has been defeated! Thanks for playing!
Stage 3.2 - Adding Items to Dungeons
In this stage, we can finally add items for the player to collect and use! Items are added in the setup phase.
Enter Command: t [dungeon_number] [item_type] [points]
dungeon_number
: which dungeon the item should be added to, indexed from 1.item_type
: theenum item_type
the new item is.points
: the point value the item is worth when used.
There are two functions to implement for Stage 3.2, create_item
and add_item
. create_item
will be similar to the create functions in Stage 1.1, where you will need to use malloc
.
In this stage you will need to create a struct item
, and add it to the dungeon indicated by dungeon_number
(dungeons indexed from 1). Items should be added in the same order as listed in the enum, i.e.:
- Physical weapons
- Magical tomes
- Armor
- Health potions
- Treasure
If there is already an item of the same type, add it to the end of that type's section, e.g. if the list of items had:
- Physical weapon
- Armor
- Treasure
A second physical weapon should be placed in the second position, becoming:
- Physical weapon
- Physical weapon (new item)
- Armor
- Treasure
Clarifications
- Refer to
cs_dungeon.h
to see what should be returned in the case of errors.
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Wizard ----------Setup Phase---------- Enter Command: a forest 2 2 forest has been added as a dungeon to the map! Enter Command: t 1 0 1 Item successfully added to the dungeon! Enter Command: t 1 1 1 Item successfully added to the dungeon! Enter Command: t 1 2 1 Item successfully added to the dungeon! Enter Command: t 1 3 1 Item successfully added to the dungeon! Enter Command: t 1 4 1 Item successfully added to the dungeon! Enter Command: q Please enter the required item to defeat the final boss: 1 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: Present Monster: Goblin Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: d ======Dungeon Details====== Minsc is currently in forest There are 2 goblins The boss is in this dungeon Health Points: 35 Damage: 10 Points: 20 Required Item: Magical Tome The dungeon forest has the following items: 1. Physical Weapon, worth 1 point(s). 2. Magical Tome, worth 1 point(s). 3. Armor, worth 1 point(s). 4. Health Potion, worth 1 point(s). 5. Treasure, worth 1 point(s). Enter Command: Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Wizard ----------Setup Phase---------- Enter Command: a forest 2 2 forest has been added as a dungeon to the map! Enter Command: t 1 1 1 Item successfully added to the dungeon! Enter Command: t 1 0 1 Item successfully added to the dungeon! Enter Command: t 1 2 1 Item successfully added to the dungeon! Enter Command: t 1 3 1 Item successfully added to the dungeon! Enter Command: t 1 1 2 Item successfully added to the dungeon! Enter Command: t 1 2 2 Item successfully added to the dungeon! Enter Command: q Please enter the required item to defeat the final boss: 1 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: Present Monster: Goblin Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: d ======Dungeon Details====== Minsc is currently in forest There are 2 Goblins The boss is in this dungeon Health Points: 35 Damage: 10 Points: 20 Required Item: Magical Tome The dungeon forest has the following items: 1. Physical Weapon, worth 1 point(s). 2. Magical Tome, worth 1 point(s). 3. Magical Tome, worth 2 point(s). 4. Armor, worth 1 point(s). 5. Armor, worth 2 point(s). 6. Health Potion, worth 1 point(s). Enter Command: Thanks for playing!
Stage 3.3 - Collecting Items
Now we would like to be able to pick up these items and place them into the player's inventory!
Enter Command: c [item_number]
The player will specify which item from the dungeon they are currently in that they would like to pick up, by the item_number
(items are indexed from 1).
The item selected should be moved from the dungeon’s list of items to the player’s list of items, inserted at the head of the player’s list of items, so that it is first in their inventory.
Point value or item usage is not added to the player’s stats (this will happen when the player uses an item).
There is one function to implement for Stage 3.3, called collect_item
. This function returns an int
; INVALID_ITEM
should be returned when the item_number
does not correspond to an item in the dungeon. Otherwise, VALID
should be returned.
Clarifications
- Refer to
cs_dungeon.h
to check what should be returned in the case of errors. INVALID
can also be returned instead ofINVALID_ITEM
.
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Wizard ----------Setup Phase---------- Enter Command: a forest 2 2 forest has been added as a dungeon to the map! Enter Command: t 1 1 1 Item successfully added to the dungeon! Enter Command: t 1 0 1 Item successfully added to the dungeon! Enter Command: t 1 2 1 Item successfully added to the dungeon! Enter Command: q Please enter the required item to defeat the final boss: 1 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: Present Monster: Goblin Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: d ======Dungeon Details====== Minsc is currently in forest There are 2 Goblins The boss is in this dungeon Health Points: 35 Damage: 10 Points: 20 Required Item: Magical Tome The dungeon forest has the following items: 1. Physical Weapon, worth 1 point(s). 2. Magical Tome, worth 1 point(s). 3. Armor, worth 1 point(s). Enter Command: s =======Player Stats======= Minsc is currently in forest Wizard Health Points: 15 Shield Power: 0 Damage: 7 Magic Modifier: 1.5 Points Collected: 0 Minsc has the following items in their inventory: No Items Enter Command: c 2 Item successfully added to Minsc's inventory! Enter Command: c 2 Item successfully added to Minsc's inventory! Enter Command: c 1 Item successfully added to Minsc's inventory! Enter Command: d ======Dungeon Details====== Minsc is currently in forest There are 2 Goblins The boss is in this dungeon Health Points: 35 Damage: 10 Points: 20 Required Item: Magical Tome The dungeon forest has the following items: No Items Enter Command: s =======Player Stats======= Minsc is currently in forest Wizard Health Points: 15 Shield Power: 0 Damage: 7 Magic Modifier: 1.5 Points Collected: 0 Minsc has the following items in their inventory: 1. Physical Weapon, worth 1 point(s). 2. Armor, worth 1 point(s). 3. Magical Tome, worth 1 point(s). Enter Command: Thanks for playing!
Stage 3.4 - Using Items
Now that we have items in the player's inventory, let's use them to make the player stronger and collect more points!
Enter Command: u [item_number]
In the use_item
function, given an item number corresponding to an item in the player’s inventory (indexed from 1):
- stats should be added to the player’s stats depending on the item and its point value.
- The item's point value should also be added to the player's collected point score.
- The item should be removed from the player’s inventory and freed.
The item effects are listed below.
Physical Weapons | Physical weapons should add to the player’s damage by their point value. |
---|---|
Magical Tome | Magical tomes should add to the player’s magic modifier by their point value divided by 10.0 |
Armor | Armor should add to the player’s shield power by their point value divided by 2. |
Health Potions | Health potions should add to the player’s health points by their point value + 5. Health cannot exceed the constant, MAX_HEALTH . |
Treasure | Treasure has no effect on the player’s stats. |
Clarifications
INVALID_ITEM
should be returned when theitem_number
does not correspond to an item in the player's inventory. Otherwise,VALID
should be returned.
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Wizard ----------Setup Phase---------- Enter Command: a forest 2 2 forest has been added as a dungeon to the map! Enter Command: t 1 1 1 Item successfully added to the dungeon! Enter Command: t 1 0 1 Item successfully added to the dungeon! Enter Command: t 1 2 1 Item successfully added to the dungeon! Enter Command: q Please enter the required item to defeat the final boss: 1 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: Present Monster: Goblin Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: s =======Player Stats======= Minsc is currently in forest Wizard Health Points: 15 Shield Power: 0 Damage: 7 Magic Modifier: 1.5 Points Collected: 0 Minsc has the following items in their inventory: No Items Enter Command: c 1 Item successfully added to Minsc's inventory! Enter Command: s =======Player Stats======= Minsc is currently in forest Wizard Health Points: 15 Shield Power: 0 Damage: 7 Magic Modifier: 1.5 Points Collected: 0 Minsc has the following items in their inventory: 1. Physical Weapon, worth 1 point(s). Enter Command: u 1 Item successfully used and removed from Minsc's inventory! Enter Command: s =======Player Stats======= Minsc is currently in forest Wizard Health Points: 15 Shield Power: 0 Damage: 8 Magic Modifier: 1.5 Points Collected: 1 Minsc has the following items in their inventory: No Items Enter Command: Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Wizard ----------Setup Phase---------- Enter Command: a forest 2 2 forest has been added as a dungeon to the map! Enter Command: t 1 1 1 Item successfully added to the dungeon! Enter Command: t 1 0 1 Item successfully added to the dungeon! Enter Command: t 1 2 1 Item successfully added to the dungeon! Enter Command: q Please enter the required item to defeat the final boss: 1 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: Present Monster: Goblin Minsc is here |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: s =======Player Stats======= Minsc is currently in forest Wizard Health Points: 15 Shield Power: 0 Damage: 7 Magic Modifier: 1.5 Points Collected: 0 Minsc has the following items in their inventory: No Items Enter Command: c 1 Item successfully added to Minsc's inventory! Enter Command: c 2 Item successfully added to Minsc's inventory! Enter Command: c 1 Item successfully added to Minsc's inventory! Enter Command: s =======Player Stats======= Minsc is currently in forest Wizard Health Points: 15 Shield Power: 0 Damage: 7 Magic Modifier: 1.5 Points Collected: 0 Minsc has the following items in their inventory: 1. Magical Tome, worth 1 point(s). 2. Armor, worth 1 point(s). 3. Physical Weapon, worth 1 point(s). Enter Command: u 2 Item successfully used and removed from Minsc's inventory! Enter Command: s =======Player Stats======= Minsc is currently in forest Wizard Health Points: 15 Shield Power: 0 Damage: 7 Magic Modifier: 1.5 Points Collected: 1 Minsc has the following items in their inventory: 1. Magical Tome, worth 1 point(s). 2. Physical Weapon, worth 1 point(s). Enter Command: Thanks for playing!
Stage 3.5 - Removing Empty Dungeons
Once a player has cleared out a dungeon and has left the dungeon, that dungeon should be removed from the map - there’s no need to go back to it! A dungeon is clear when there are no monsters (including final bosses), no items, and the player is not in it.
You will need to add this to your end_turn
function. At this point in the assignment, a turn is as follows:
- Player action
- Monster attacks
- Remove any empty dungeons
- Check if the game is over
Examples
dcc --leak-check cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Wizard ----------Setup Phase---------- Enter Command: a forest 2 2 forest has been added as a dungeon to the map! Enter Command: t 1 1 1 Item successfully added to the dungeon! Enter Command: t 1 0 1 Item successfully added to the dungeon! Enter Command: t 1 2 1 Item successfully added to the dungeon! Enter Command: a beach 3 1 beach has been added as a dungeon to the map! Enter Command: a city 1 5 city has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 1 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: None Monster: Goblin Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. beach Boss: None Monster: Skeleton Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 3. city Boss: Present Monster: Slime Empty |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: c 1 Item successfully added to Minsc's inventory! Enter Command: c 1 Item successfully added to Minsc's inventory! Enter Command: c 1 Item successfully added to Minsc's inventory! Enter Command: u 2 Item successfully used and removed from Minsc's inventory! Enter Command: # A battle has raged! Enter Command: > Moved into the next dungeon Enter Command: # A battle has raged! Enter Command: p Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. beach Boss: None Monster: Skeleton Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. city Boss: Present Monster: Slime Empty |^|^|^|^|^| |^|^|^|^|^| Enter Command: > Moved into the next dungeon Enter Command: p Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. city Boss: Present Monster: Slime Minsc is here |^|^|^|^|^| |^|^|^|^|^| Enter Command: Thanks for playing!
Testing and Submission
Remember to do your own testing
Are you finished with this stage? If so, you should make sure to do the following:
- Run
1511 style
and clean up any issues a human may have reading your code. Don't forget -- 20% of your mark in the assignment is based on style and readability! - Autotest for this stage of the assignment by running the
autotest-stage
command as shown below. - Remember -- give early and give often. Only your last submission counts, but why not be safe and submit right now?
1511 style cs_dungeon.c 1511 autotest-stage 03 cs_dungeon give cs1511 ass2_cs_dungeon cs_dungeon.c
Stage 4
This stage is for students who want to challenge themselves, and solve more complicated linked lists and programming problems, such as:
- Teleportation between dungeons
- Defeating the final boss
Stage 4.1 - Teleportation Between Dungeons
In Stage 4.1, we’d like to be able to teleport the player between dungeons.
Enter Command: T
All dungeons can be teleported to. When the T
command is entered, the player should teleport to the furthest dungeon from the current dungeon they are in, unless that dungeon has already been teleported to. Once all dungeons have been teleported to, the pattern can begin again.
For example, a map with 4 dungeons. If the player starts in the first dungeon, their movements would be as follows (the player is represented by the knight character):
There is one function in cs_dungeon.c
that you will have to implement for Stage 4.1, teleport
. INVALID
should be returned when there is only one dungeon in the map, otherwise, VALID
should be returned.
Clarifications
- Whenever the map is changed (by shuffling in Stage 4.2 or by removing empty dungeons), the teleportation movement should restart, as if no dungeons have been teleported to yet.
- When the player moves between dungeons with
>
or<
, the teleportation movement should restart, as if no dungeons have been teleported to yet. - If multiple dungeons are equidistant, the player should travel to the first one in the map.
- The first dungeon the player starts the teleportation cycle in counts as being teleported to.
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a forest 1 1 forest has been added as a dungeon to the map! Enter Command: a beach 2 2 beach has been added as a dungeon to the map! Enter Command: a castle 3 3 castle has been added as a dungeon to the map! Enter Command: a city 1 5 city has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 1 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: None Monster: Slime Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. beach Boss: None Monster: Goblin Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 3. castle Boss: None Monster: Skeleton Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 4. city Boss: Present Monster: Slime Empty |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: T Minsc has teleported! Enter Command: p Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: None Monster: Slime Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. beach Boss: None Monster: Goblin Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 3. castle Boss: None Monster: Skeleton Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 4. city Boss: Present Monster: Slime Minsc is here |^|^|^|^|^| |^|^|^|^|^| Enter Command: T Minsc has teleported! Enter Command: p Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: None Monster: Slime Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. beach Boss: None Monster: Goblin Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 3. castle Boss: None Monster: Skeleton Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 4. city Boss: Present Monster: Slime Empty |^|^|^|^|^| |^|^|^|^|^| Enter Command: T Minsc has teleported! Enter Command: p Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: None Monster: Slime Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. beach Boss: None Monster: Goblin Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 3. castle Boss: None Monster: Skeleton Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 4. city Boss: Present Monster: Slime Empty |^|^|^|^|^| |^|^|^|^|^| Enter Command: T Minsc has teleported! Enter Command: p Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: None Monster: Slime Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. beach Boss: None Monster: Goblin Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 3. castle Boss: None Monster: Skeleton Empty |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 4. city Boss: Present Monster: Slime Empty |^|^|^|^|^| |^|^|^|^|^| Enter Command: Thanks for playing!
Stage 4.2 - The Final Boss
We have finally reached the final boss! When the final boss is attacked for the first time, it becomes so enraged, spreading its power across the dungeon, shaking things up so that the map becomes unrecognisable. In this stage you will be implementing the boss_fight
function.
Enter Command: b
To be able to attack the final boss, the player must have the required item type in their inventory, not yet used.
When the player attacks the final boss, they should deal either physical damage or magical damage, as calculated in fight
from Stage 2.4. They should do whichever does more damage. If the damage is equal, they should deal magical damage.
The first time the boss is attacked, the map should be shuffled, so that pairs of dungeons switch places. Below is an example diagram of a dungeon map containing 4 dungeons:
When attacking a boss, the boss can sustain damage, and can be damaged across multiple turns. Bosses will fight back each turn the player is in the same dungeon, and when they reach 50% of their original health or less, they will do 1.5 times more damage.
Bosses will follow the player (but cannot teleport), and will attempt to reach the player by moving towards them each turn. If they are in the same dungeon as the player, they will attack. Bosses can move or attack, but not both in the same turn.
You will need to add code to your end_turn
function. A turn should run as follows:
- Player action (including shuffling the map if it is the first time the boss is attacked)
- Monster attacks
- Remove any empty dungeons
- Boss attack or move
- Check if the game is over
If the player defeats the boss, then you should call the helper function print_boss_defeat
after both the player and the boss have done their actions for the turn.
Clarifications
- If there is an odd number of dungeons, e.g. 5 dungeons, swap the first 4, leaving the last dungeon in its original position.
boss_fight
should returnNO_BOSS
when there is no boss present in the current dungeon,NO_ITEM
when the player does not have the required item to fight the boss, andVALID
otherwise.- Make sure to go back and check your code from Stage 3.1 to check if the boss has been defeated. If the boss is defeated, their points should be added to the player's point total.
- Whenever the map is changed, the teleportation movement Stage 4.1 should restart, as if no dungeons have been teleported to yet.
- The boss only start moving once attacked for the first time.
- You may assume the same shield power logic as Stage 2.4 applies to the boss damage.
Examples
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a forest 1 1 forest has been added as a dungeon to the map! Enter Command: a beach 2 2 beach has been added as a dungeon to the map! Enter Command: q Please enter the required item to defeat the final boss: 1 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: None Monster: Slime Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. beach Boss: Present Monster: Goblin Empty |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: b ERROR: There is no boss in this dungeon! Enter Command: > Moved into the next dungeon Enter Command: b ERROR: Minsc does not have the required item to defeat the boss! Enter Command: Thanks for playing!
dcc cs_dungeon.c main.c -o cs_dungeon ./cs_dungeon Welcome to the 1511 Dungeon! This is a game where you get to create your own dungeon map, battle monsters and collect items! Please enter the name of your map: Faerun Please enter the amount of points required to win: 25 Please enter the player's name: Minsc Player Class Options: Fighter Wizard Please enter player's chosen class type: Fighter ----------Setup Phase---------- Enter Command: a forest 1 1 forest has been added as a dungeon to the map! Enter Command: a beach 2 2 beach has been added as a dungeon to the map! Enter Command: t 1 1 1 Item successfully added to the dungeon! Enter Command: t 1 0 5 Item successfully added to the dungeon! Enter Command: t 1 0 5 Item successfully added to the dungeon! Enter Command: t 1 3 5 Item successfully added to the dungeon! Enter Command: t 1 3 5 Item successfully added to the dungeon! Enter Command: q Please enter the required item to defeat the final boss: 1 The final boss has been added to Faerun! Map of Faerun! |^|^|^|^|^| |^|^|^|^|^| 1. forest Boss: None Monster: Slime Minsc is here |^|^|^|^|^| |^|^|^|^|^| | | | | | | |^|^|^|^|^| |^|^|^|^|^| 2. beach Boss: Present Monster: Goblin Empty |^|^|^|^|^| |^|^|^|^|^| --------Gameplay Phase-------- Enter Command: c 1 Item successfully added to Minsc's inventory! Enter Command: c 1 Item successfully added to Minsc's inventory! Enter Command: c 1 Item successfully added to Minsc's inventory! Enter Command: c 1 Item successfully added to Minsc's inventory! Enter Command: c 1 Item successfully added to Minsc's inventory! Enter Command: u 1 Item successfully used and removed from Minsc's inventory! Enter Command: u 1 Item successfully used and removed from Minsc's inventory! Enter Command: u 2 Item successfully used and removed from Minsc's inventory! Enter Command: u 2 Item successfully used and removed from Minsc's inventory! Enter Command: s =======Player Stats======= Minsc is currently in forest Fighter Health Points: 50 Shield Power: 2 Damage: 18 Magic Modifier: 0.9 Points Collected: 20 Minsc has the following items in their inventory: 1. Magical Tome, worth 1 point(s). Enter Command: > Moved into the next dungeon Enter Command: b A battle has raged against the dungeon boss! Enter Command: s =======Player Stats======= Minsc is currently in beach Fighter Health Points: 37 Shield Power: 2 Damage: 18 Magic Modifier: 0.9 Points Collected: 20 Minsc has the following items in their inventory: 1. Magical Tome, worth 1 point(s). Enter Command: b A battle has raged against the dungeon boss! The boss has been defeated! Minsc the Fighter has won by collecting 25 points and defeating the final boss! Thanks for playing!
Testing and Submission
Remember to do your own testing
Are you finished with this stage? If so, you should make sure to do the following:
- Run
1511 style
and clean up any issues a human may have reading your code. Don't forget -- 20% of your mark in the assignment is based on style and readability! - Autotest for this stage of the assignment by running the
autotest-stage
command as shown below. - Remember -- give early and give often. Only your last submission counts, but why not be safe and submit right now?
1511 style cs_dungeon.c 1511 autotest-stage 04 cs_dungeon give cs1511 ass2_cs_dungeon cs_dungeon.c
Extension
As an extension, we have set up a starting point to add a texture pack to your game via splashkit.
Extension activities are not work any marks, nor are there any autotests.
Installing Splashkit
To install splashkit, run the following command
1511 setup-splashkit cs_dungeon
Check that Splashkit Works
Lets check that splashkit works by running a test file. It should display a white square with a grid inside.
The previous command should have added splashkit_example.c
to your current
directory. If you can't find it, run: cp -n /web/cs1511/24T3/activities/cs_dungeon/splashkit_example.c .
.
skm clang++ splashkit_example.c -o splashkit_example ./splashkit_example
Running your code with splashkit
The last two commands are a bit like your new dcc cs_dungeon.c -o cs_dungeon
and ./cs_dungeon
but for splashkit. So it will look a bit like:
skm clang++ cs_dungeon.c -o cs_dungeon ./cs_dungeon
Starting Points for Splashkit
Have a look inside of splashkit_example.c
to see what they have added. A
couple things to note are:
#include "splashkit.h"
is needed at the top of the file.- Line 48,
window w = open_window("Splashkit Example", 600, 400);
needs to be run when you decide to open your viewable window. - The loop in
main
allows us to update the screen according to any user events, and refreshes the screen after anything is drawn on the frame.
Expanding CS Dungeon
Now that you have completed our version of CS Dungeon, you can expand on it to create your own version! You can add more class types, monsters, bosses, commands - anything you want!
Make sure that you have submitted cs_dungeon.c
before changing it, as extension activities are not worth any marks, and are not autotested.
First, create a copy of your current files, where you can start your version, like this:
mkdir cs_dungeon_expanded cp main.c cs_dungeon.h cs_dungeon.c cs_dungeon_expanded cd cs_dungeon_expanded ls cs_dungeon.c cs_dungeon.h main.c
Now, you can edit both cs_dungeon.c
and main.c
to accomdate for any new commands you create!
Here are some ideas of things you can add to get you started:
- new classes (you can take inspiration from the traditional Dungeons and Dragons classes, like cleric, warlock, barbarian, etc.)
- new monsters
- new final boss monsters with different stats, and different shuffling abilities (e.g. alphabetical)
- more complicated attacks and class powers
- more detailed dungeons, like adding terrain that can affect the player and monsters (making them stronger or weaker)
- branching dungeons - instead of each dungeon leading to one more, what if each dungeon could lead to 2 more? Or 3? Or 4?
- more items with new abilities
Tools
Creating Dungeons in Separate Files
If you are getting sick of typing in your inputs for a dungeon every single time you run CS Dungeon, you might want to store your input in a separate file. This allows you to see the result of the setup phase or to play your dungeon straight away.
1. Create a file for your input.
First, let's create a file to store in the input for a dungeon that you have created. This isn't a .c
file, its just a regular plain text file, the file extension .in
works nicely for this!
Let's put it in the same directory as your cs_dungeon.c code.
ls main.c cs_dungeon.h cs_dungeon.c cs_dungeon my_dungeon.in
2. Add your input to the file
Inside of this file, add the input for the dungeon. Don't add in any of the gameplay commands as those will come from the terminal.
Your file could look a bit like this (including the newline at the end):
Faerun 25 Minsc Wizard a forest 3 3 a beach 1 2 q 2
3. Run the code with your file
Next, instead of just running ./cs_dungeon
, lets tell the computer to first read from the file we created.
If you have completed stage 2.2 then use the following:
cat my_dungeon.in - | ./cs_dungeon
Otherwise, use the following:
cat my_dungeon.in | ./cs_dungeon
This will also work on the reference implementation too!
If you have completed stage 2.2 then use the following:
cat my_dungeon.in - | 1511 cs_dungeon
Otherwise, use the following:
cat my_dungeon.in | 1511 cs_dungeon
Assessment
Assignment Conditions
-
Joint work is not permitted on this assignment.
This is an individual assignment.
The work you submit must be entirely your own work. Submission of any work even partly written by any other person is not permitted.
The only exception being if you use small amounts (< 10 lines) of general purpose code (not specific to the assignment) obtained from a site such as Stack Overflow or other publicly available resources. You should attribute the source of this code clearly in an accompanying comment.
Assignment submissions will be examined, both automatically and manually for work written by others.
Do not request help from anyone other than the teaching staff of COMP1511.
Do not post your assignment code to the course forum - the teaching staff can view assignment code you have recently autotested or submitted with give.
Rationale: this assignment is an individual piece of work. It is designed to develop the skills needed to produce an entire working program. Using code written by or taken from other people will stop you learning these skills.
-
The use of code-synthesis tools, such as GitHub Copilot, is not permitted on this assignment.
The use of Generative AI to generate code solutions is not permitted on this assignment.
Rationale: this assignment is intended to develop your understanding of basic concepts. Using synthesis tools will stop you learning these fundamental concepts.
-
Sharing, publishing, distributing your assignment work is not permitted.
Do not provide or show your assignment work to any other person, other than the teaching staff of COMP1511. For example, do not share your work with friends.
Do not publish your assignment code via the internet. For example, do not place your assignment in a public GitHub repository.
Rationale: by publishing or sharing your work you are facilitating other students to use your work, which is not permitted. If they submit your work, you may become involved in an academic integrity investigation.
-
Sharing, publishing, distributing your assignment work after the completion of COMP1511 is not permitted.
For example, do not place your assignment in a public GitHub repository after COMP1511 is over.
Rationale:COMP1511 sometimes reuses assignment themes, using similar concepts and content. If students in future terms can find your code and use it, which is not permitted, you may become involved in an academic integrity investigation.
Violation of the above conditions may result in an academic integrity investigation with possible penalties, up to and including a mark of 0 in COMP1511 and exclusion from UNSW.
Relevant scholarship authorities will be informed if students holding scholarships are involved in an incident of plagiarism or other misconduct. If you knowingly provide or show your assignment work to another person for any reason, and work derived from it is submitted - you may be penalised, even if the work was submitted without your knowledge or consent. This may apply even if your work is submitted by a third party unknown to you.
If you have not shared your assignment, you will not be penalised if your work is taken without your consent or knowledge.
For more information, read the UNSW Student Code , or contact the course account. The following penalties apply to your total mark for plagiarism:
0 for the assignment | Knowingly providing your work to anyone and it is subsequently submitted (by anyone). |
0 for the assignment | Submitting any other person's work. This includes joint work. |
0 FL for COMP1511 | Paying another person to complete work. Submitting another person's work without their consent. |
Submission of Work
You should submit intermediate versions of your assignment. Every time you
autotest or submit, a copy will be saved as a backup. You can find those
backups
here
, by logging in, and choosing the yellow button next to
ass2_cs_dungeon
.
Every time you work on the assignment and make some progress, you should copy
your work to your CSE account and submit it using the give
command below.
It is fine if intermediate versions do not compile or otherwise fail submission tests.
Only the final submitted version of your assignment will be marked.
You submit your work like this:
give cs1511 ass2_cs_dungeon cs_dungeon.c
Assessment Scheme
This assignment will contribute 25% to your final mark.
80% of the marks for this assignment will be based on the performance of the
code you write in cs_dungeon.c
.
20% of the marks for this assignment will come from manual marking of the readability of the C you have written. The manual marking will involve checking your code for clarity, and readability, which includes the use of functions and efficient use of loops and if statements.
Marks for your performance will be allocated roughly according to the below scheme.
COMP1911
100% for Performance | Completely working implementation of Stage 1 and Stage 2. |
55% for Performance | Completely working implementation of Stage 1. |
COMP1511
100% for Performance | Completely Working Implementation, which exactly follows the specification (Stage 1, 2, 3 and 4). |
85% for Performance | Completely working implementation of Stage 1, 2 and 3. |
65% for Performance | Completely working implementation of Stage 1 and Stage 2. |
35% for Performance | Completely working implementation of Stage 1. |
Marks for your style will be allocated roughly according to the scheme below.
Style Marking Rubric
0 | 1 | 2 | 3 | 4 | |
Formatting (/5) | |||||
Indentation (/2) - Should use a consistent indentation scheme. | Multiple instances throughout code of inconsistent/bad indentation | Code is mostly correctly indented | Code is consistently indented throughout the program | ||
Whitespace (/1) - Should use consistent whitespace (for example, 3 + 3 not 3+ 3) | Many whitespace errors | No whitespace errors | |||
Vertical Whitespace (/1) - Should use consistent whitespace (for example, vertical whitespace between sections of code) | Code has no consideration for use of vertical whitespace | Code consistently uses reasonable vertical whitespace | |||
Line Length (/1) - Lines should be max. 80 characters long | Many lines over 80 characters | No lines over 80 characters | |||
Documentation (/5) | |||||
Comments (incl. header comment) (/3) - Comments have been used throughout the code above code sections and functions to explain their purpose. A header comment (with name, zID and a program description) has been included | No comments provided throughout code | Few comments provided throughout code | Comments are provided as needed, but some details or explanations may be missing causing the code to be difficult to follow | Comments have been used throughout the code above code sections and functions to explain their purpose. A header comment (with name, zID and a program description) has been included | |
Function/variable/constant naming (/2) - Functions/variables/constants names all follow naming conventions in style guide and help in understanding the code | Functions/variables/constants names do not follow naming conventions in style guide and help in understanding the code | Functions/variables/constants names somewhat follow naming conventions in style guide and help in understanding the code | Functions/variables/constants names all follow naming conventions in style guide and help in understanding the code | ||
Organisation (/5) | |||||
Function Usage (/4) - Code has been decomposed into appropriate functions separating functionalities | No functions are present, code is one main function | Some code has been moved to functions | Some code has been moved to sensible/thought out functions, and/or many functions exceed 50 lines (incl. main function) | Most code has been moved to sensible/thought out functions, and/or some functions exceed 50 lines (incl. main function) | All code has been meaningfully decomposed into functions of a maximum of 50 lines (incl. The main function) |
Function Prototypes (/1) - Function Prototypes have been used to declare functions above main | Functions are used but have not been prototyped | All functions have a prototype above the main function or no functions are used | |||
Elegance (/5) | |||||
Overdeep nesting (/2) - You should not have too many levels of nesting in your code (nesting which is 5 or more levels deep) | Many instances of overdeep nesting | <= 3 instances of overdeep nesting | No instances of overdeep nesting | ||
Code Repetition (/2) - Potential repetition of code has been dealt with via the use of functions or loops | Many instances of repeated code sections | <= 3 instances of repeated code sections | Potential repetition of code has been dealt with via the use of functions or loops | ||
Constant Usage (/1) - Any magic numbers are #defined | None of the constants used throughout program are #defined | All constants used are #defined and are used consistently in the code | |||
Illegal elements | |||||
Illegal elements - Presence of any illegal elements indicated in the style guide | CAP MARK AT 16/20 |
Note that the following penalties apply to your total mark for plagiarism:
0 for the assignment | Knowingly providing your work to anyone and it is subsequently submitted (by anyone). |
0 for the assignment | Submitting any other person's work. This includes joint work. |
0 FL for COMP1511 | Paying another person to complete work. Submitting another person's work without their consent. |
Allowed C Features
In this assignment, there are no restrictions on C Features, except for those in the style guide. If you choose to disregard this advice, you must still follow the style guide.
You also may be unable to get help from course staff if you use features not taught in COMP1511. Features that the Style Guide identifies as illegal will result in a penalty during marking. You can find the style marking rubric above. Please note that this assignment must be completed using only Linked Lists . Do not use arrays in this assignment. If you use arrays instead of lined lists you will receive a 0 for performance in this assignment.
Due Date
This assignment is due 15 November 2024 17:00:00. For each day after that time, the maximum mark it can achieve will be reduced by 5% (off the ceiling).- For instance, at 1 day past the due date, the maximum mark you can get is 95%.
- For instance, at 3 days past the due date, the maximum mark you can get is 85%.
- For instance, at 5 days past the due date, the maximum mark you can get is 75%.
Change Log
-
Version 1.0
(2024-10-24 13:00) -
- Assignment Released
-
Version 1.1
(2024-10-24 19:00) -
- Fixed fighter damage in stage 1 testing file expected output to match the specification.
-
Version 1.2
(2024-10-26 16:00) -
- Added clarification to 2.4 regarding when powers can be used and fixed numbering on monster type error message
-
Version 1.3
(2024-10-27 13:40) -
- Fixed reference solution for 4.2 not adding points after defeating the final boss and infinite-health bug in bosses.
-
Version 1.4
(2024-10-20 15:45) -
- Fixed typo in example 2.1.2.
-
Version 1.5
(2024-11-2 12:11) -
- Fixed typos in example 2.4.2.
-
Version 1.6
(2024-11-2 23:02) -
- Fixed typos in examples 2.1.2 and 2.1.3
-
Version 1.7
(2024-11-2 23:10) -
- Fixed typo in example 2.1.4
-
Version 1.8
(2024-11-4 19:22) -
- Fixed typo in example 1.5.1